Some of the CSS properties are widely used to apply style on HTML tables. Each of them is described below.
In this chapter, we will talk about how to give styles to tables. We can change the color of headings and rows that we want.
Table Styling Properties
Here are CSS properties that we use for applying a style to the table. The background-color and color properties set the background color and the color of the text, respectively. The border-collapse property makes the table borders collapse. The text-align property sets the text position. Also, we should use the height, width and padding properties for styling.
Example of styling a table:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
table {
width: 100%;
border-collapse: collapse;
}
table,
th,
td {
border: 1px solid black;
}
thead {
background-color: #1c87c9;
color: #ffffff;
}
th {
text-align: center;
height: 50px;
}
tbody tr:nth-child(odd) {
background: #ffffff;
}
tbody tr:nth-child(even) {
background: #f4f4f4;
}
</style>
</head>
<body>
<table>
<thead>
<tr>
<th>Heading</th>
<th>Heading</th>
</tr>
</thead>
<tbody>
<tr>
<td>Some text</td>
<td>Some text</td>
</tr>
<tr>
<td>Some text</td>
<td>Some text</td>
</tr>
<tr>
<td>Some text</td>
<td>Some text</td>
</tr>
<tr>
<td>Some text</td>
<td>Some text</td>
</tr>
</tbody>
</table>
</body>
</html>
Result
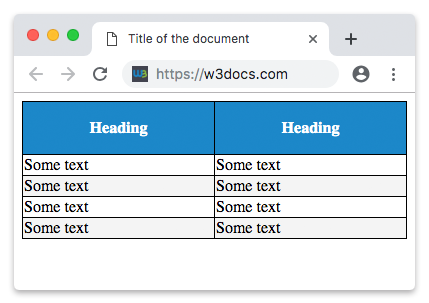
Let's explain the above code.
As you see our table has 2 parts: the first is the part where we have written headings, which is our <thead> part and the second part is the <tbody> part where we have written some text. The table has black borders, for which we use border property. We can use any color we want as well as we can choose the style of borders.
Table color
As you see the <thead> part of our table is blue and wherever we write some text is in white. For the blue background, we use the background-color property, and for the white text, we use the color property. The other texts are written with black.
Collapse Borders
The border-collapse property specifies whether the borders of a table are collapsed into a single border or separated.
Table Width and Height
The table also has width and height properties. As you see we use these properties in our style. We use the width property for the whole table and the height property for the headings. We can use these properties with pixels and percents.
Table Text Alignment
Now about the table text alignment. As you know earlier we used the text-align property for the text position. In our example, as you see, we use the text-align property for the heading. For that, we use "text-align: center". You can read our previous chapter to know how to use it.
Table Padding
To control the space between the border and content in a table, use the padding property on <td> and <th> elements:
td {
padding: 15px;
}
Horizontal alignment with the text-align property
With the help of the CSS text-align property, you can set the horizontal alignment of the content in <th> or <td>.
By default, the content of <td> elements are aligned to the left and the content of the <th> elements are aligned to the center. In the example below, the content of <th> and <td> elements is aligned to the right:
Example of aligning the content of <th> and <td> elements to the right:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
table,
td,
th {
border: 1px solid black;
}
table {
border-collapse: collapse;
width: 100%;
}
th,
td {
text-align: right;
}
</style>
</head>
<body>
<h2>Horizontal alignment example</h2>
<table>
<tbody>
<tr>
<th>Firstname</th>
<th>Lastname</th>
<th>Money</th>
</tr>
<tr>
<td>Sherlock</td>
<td>Holmes</td>
<td>$200</td>
</tr>
<tr>
<td>John</td>
<td>Watson</td>
<td>$250</td>
</tr>
<tr>
<td>Mary</td>
<td>Whatson</td>
<td>$500</td>
</tr>
<tbody>
</table>
</body>
</html>
Vertical alignment with the vertical align-property
Using the CSS vertical-align property, you can set the vertical alignment of the content in <th> or <td>.
By default, the content in both <th> and <td> elements is vertically aligned to middle.
In the example below, the content of <td> elements is vertically aligned to bottom:
Example of the vertical alignment of <td> elements’ content to bottom:
<!DOCTYPE html>
<html>
<head>
<style>
table,
td,
th {
border: 1px solid black;
}
table {
border-collapse: collapse;
width: 100%;
}
td {
height: 50px;
vertical-align: bottom;
text-align: right;
padding-right: 10px;
}
</style>
</head>
<body>
<h2>Vertical alignment example</h2>
<table>
<tr>
<th>Firstname</th>
<th>Lastname</th>
<th>Money</th>
</tr>
<tr>
<td>Sherlock</td>
<td>Holmes</td>
<td>$300</td>
</tr>
<tr>
<td>John</td>
<td>Watson</td>
<td>$250</td>
</tr>
<tr>
<td>Mary</td>
<td>Watson</td>
<td>$500</td>
</tr>
</table>
</body>
</html>
Horizontal dividers
By adding the CSS border-bottom property to <td> and <th> elements, you will create horizontal dividers.
Example of creating horizontal dividers:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
table {
border-collapse: collapse;
width: 100%;
}
th,
td {
padding: 10px;
text-align: left;
border-bottom: 1px solid #cccccc;
}
</style>
</head>
<body>
<h2>Horizontal dividers example</h2>
<table>
<tr>
<th>Firstname</th>
<th>Lastname</th>
<th>Money</th>
</tr>
<tr>
<td>Sherlock</td>
<td>Holmes</td>
<td>$200</td>
</tr>
<tr>
<td>John</td>
<td>Watson</td>
<td>$350</td>
</tr>
<tr>
<td>Mary</td>
<td>Watson</td>
<td>$500</td>
</tr>
</table>
</body>
</html>
Hoverable tables
Using the CSS :hover selector on <tr> element, will make the table hoverable.
Example of creating a hoverable table:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
table {
border-collapse: collapse;
width: 100%;
}
tr {
background-color: #f5f5f5;
}
th,
td {
padding: 15px;
text-align: left;
border-bottom: 1px solid #ccc;
}
tr:hover {
background-color: #cdcdcd;
}
</style>
</head>
<body>
<h2>Hoverable table example</h2>
<table>
<tr>
<th>First Name</th>
<th>Last Name</th>
<th>Money</th>
</tr>
<tr>
<td>Sherlock</td>
<td>Holmes</td>
<td>$200</td>
</tr>
<tr>
<td>John</td>
<td>Watson</td>
<td>$350</td>
</tr>
<tr>
<td>Mary</td>
<td>Watson</td>
<td>$500</td>
</tr>
</table>
</body>
</html>
Zebra-striped table
Using the nth-child() selector and adding the CSS background-color property to the odd (even) table rows, you can create a zebra-striped table.
Example of creating a zebra striped table:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
table {
border-collapse: collapse;
width: 100%;
}
th,
td {
text-align: left;
padding: 10px;
}
tr:nth-child(even) {
background-color: #6eeccf;
}
tr:nth-child(odd) {
background-color: #2d7f88;
}
</style>
</head>
<body>
<h2>Striped table example</h2>
<table>
<tr>
<th>First name</th>
<th>Last name</th>
<th>Points</th>
</tr>
<tr>
<td>Sherlock</td>
<td>Holmes</td>
<td>$250</td>
</tr>
<tr>
<td>John</td>
<td>Watson</td>
<td>$350</td>
</tr>
<tr>
<td>Mary</td>
<td>Watson</td>
<td>$500</td>
</tr>
</table>
</body>
</html>
Responsive tables
Adding any container element with the "auto" value of the CSS overflow-x property to the <table> element, you can make the table responsive.
Example of creating a responsive table:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
div {
overflow-x: auto;
}
table {
border-collapse: collapse;
width: 100%;
}
th,
td {
text-align: left;
padding: 8px 5px;
}
tr:nth-child(even) {
background-color: #6eeccf;
}
tr:nth-child(odd) {
background-color: #2d7f88;
}
</style>
</head>
<body>
<h2>Responsive table example</h2>
<div>
<table>
<tr>
<th>First Name</th>
<th>Last Name</th>
<th>Money</th>
<th>Money</th>
<th>Money</th>
<th>Money</th>
<th>Money</th>
<th>Money</th>
<th>Money</th>
<th>Money</th>
<th>Money</th>
<th>Money</th>
</tr>
<tr>
<td>Sherlock</td>
<td>Holmes</td>
<td>$150</td>
<td>$150</td>
<td>$150</td>
<td>$150</td>
<td>$150</td>
<td>$150</td>
<td>$150</td>
<td>$150</td>
<td>$150</td>
<td>$150</td>
</tr>
<tr>
<td>John</td>
<td>Watson</td>
<td>$350</td>
<td>$350</td>
<td>$350</td>
<td>$350</td>
<td>$350</td>
<td>$350</td>
<td>$350</td>
<td>$350</td>
<td>$350</td>
<td>$350</td>
</tr>
<tr>
<td>Mary</td>
<td>Watson</td>
<td>$500</td>
<td>$500</td>
<td>$500</td>
<td>$500</td>
<td>$500</td>
<td>$500</td>
<td>$500</td>
<td>$500</td>
<td>$500</td>
<td>$500</td>
</tr>
</table>
</div>
</body>
</html>
Practice Your Knowledge
Quiz Time: Test Your Skills!
Ready to challenge what you've learned? Dive into our interactive quizzes for a deeper understanding and a fun way to reinforce your knowledge.