The @media at-rule specifies a set of styles that are applied only to certain media types.
Media queries are a popular technique for delivering a responsive web design to desktops, laptops, tablets, and mobile phones.
Besides media types, there are media features which have names and accept certain values like properties. But there are differences between properties and media features:
- Properties cannot display without values, and features do not require values.
- Features accept only single value unlike properties.
Media queries are used to check the following:
- width and height of the viewport
- width and height of the device
- orientation
- resolution
In JavaScript, the CSSMediaRule interface which represents a single @media rule can access the rules created with @media.
Initial Value | all |
Applies to | Certain media types. |
Inherited | No. |
Animatable | Yes. |
Version | Media Queries, CSS2 |
DOM Syntax | object.style.@media = "screen and (min-width: 500px)"; |
Syntax
@media: media-type (and media-query-feature);
Example of the @media rule:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
body {
background-color: #1c87c9;
}
@media screen and (max-width: 411px) {
body {
background-color: #cce5ff;
}
}
@media screen and (min-width: 768px) {
body {
background-color: #eee;
}
}
@media screen and (max-width: 962px) and (min-width: 450px),
(min-width: 1366px) {
background-color: #333;
}
</style>
</head>
<body>
<h2>@media rule example</h2>
<p>Resize the browser to see the effect.</p>
<p>Media queries set the background-color to light gray if the viewport is 600 pixels wide or wider, to green if the viewport is between 200 and 599 pixels wide. If the viewport is smaller than 200 pixels, the background-color is blue.</p>
</body>
</html>
Result
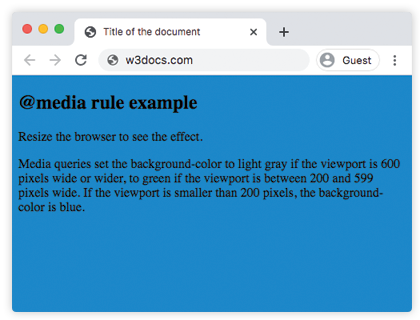
In the following example, when the browser's width is between 500 and 800px or above 1000px, the appearance of <div> changes. Resize the browser window to see the effect.
Example of the @media rule with a changed appearance of <div>:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
body {
background-color: #1c87c9;
}
@media screen and (min-width: 200px) {
body {
background-color: #8ebf42;
}
}
@media screen and (min-width: 600px) {
body {
background-color: #ccc;
}
}
@media screen and (max-width: 800px) and (min-width: 500px),
(min-width: 1000px) {
div.box {
font-size: 50px;
padding: 50px;
border: 8px solid #000;
background: #eee;
}
}
</style>
</head>
<body>
<h2>@media rule example</h2>
<p>Mediaqueries sets the background-color to grey if the viewport is 600 pixels wide or wider, to green if the viewport is between 200 and 599 pixels wide. If the viewport is smaller than 200 pixels, the background-color is blue.</p>
<h3>Change the appearance of DIV on different screen sizes</h3>
<div class="box">DIV</div>
</body>
</html>
Media Types
Value | Description |
---|---|
all | This value is used for all devices. This is the default value of this property. |
Is used for printers. | |
screen | Is used for color computer screens. |
speech | Is used for speakers. |
initial | Makes the property use its default value. |
inherit | Inherits the property from its parents element. |
Example of using media for hiding an element on extra small devices:
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1, user-scalable=no">
<style>
@media (max-width: 767px) {
.hide-mobile {
display: none;
}
}
</style>
</head>
<body>
<h1>Hi</h1>
<p>There is some text for example.</p>
<p class="hide-mobile">This text will be hidden on large devices.</p>
<p>There is some text for example.</p>
</body>
</html>
Example of using media for changing the content background color on different devices.
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
@media screen and (max-width: 767px) {
.content {
background-color: lightblue;
padding: 30px;
}
}
@media screen and (min-width: 768px) {
.content {
background-color: pink;
padding: 10px;
}
}
@media screen and (min-width: 800px) {
.content {
background-color: lightgreen;
color: white;
padding: 50px;
}
}
</style>
</head>
<body>
<div class="content">
<h2>Resize the browser to see the effect.</h2>
<p>
CSS is a rule-based language, which means that you define
rules specifying groups of styles applying to particular
elements or groups of elements on the web page.
</p>
</div>
</body>
</html>
Media Features
Value | Description |
---|---|
width | The width of the viewport. css2 |
height | The height of the viewport. |
orientation | The orientation of the viewport. |
scan | The scanning of the viewport. |
aspect-ratio | The ratio of the value of the "width" media feature to the value of the "height" media feature. |
grid | Queries whether the output device is grid or bitmap. |
color | The number of bits per color component of the output device. If device is not a color one, the value is 0. |
color-gamut | The approximate range of colors that are supported by the user agent and output device. |
color-index | Defines the number of entries in the color lookup table of the output device. If the device does not use color lookup, the value is 0. |
inverted-colors | Queries whether the browser or underlying OS invert colors or not. |
hover | Queries whether the primary input mechanism allows the user to hover over elements or not. |
any-hover | Queries whether the available input mechanism allows the user to hover over elements or not. |
any-pointer | Queries whether the available input mechanism is a pointing device or not. |
light-level | The light level of surroundings. |
max-aspect-ratio | The maximum ratio between the width and the height of the display area. |
max-color | The maximum number of bits per color component for the output device. |
max-color-index | The maximum number of colors for displaying. |
max-height | The maximum height of the display area. |
max-monochrome | The maximum number of bits per color on a monochrome device. |
max-resolution | The maximum resolution of the device. |
max-width | The maximum width of the display area. |
min-aspect-ratio | The minimum ratio between the width and the height of the display area. |
min-color | The minimum number of bits per color component for the output device. |
min-color-index | The minimum number of colors for displaying. |
min-height | The minimum height of the display area. |
min-monochrome | The minimum number of bits per color on a monochrome device. |
min-resolution | The minimum resolution of the device. |
min-width | The minimum width of the display area. |
monochrome | Defines the number of bits per pixel in a monochrome frame buffer. If the device is not a monochrome one, the value is 0. |
orientation | The orientation of the viewport. |
overflow-block | Queries how the output device handles content that overflows the viewport along the block axis. |
overflow-inline | Queries whether the content that overflows the viewport along the inline axis can be scrolled or not. |
pointer | Queries whether the primary input mechanism is a pointing device or not. |
resolution | Describes the resolution of the output device. |
scripting | Queries whether the scripting available or not. |
update | Queries how quickly the output device can modify the appearance of the content. |
Browser support
|
|
|
|
|
---|---|---|---|---|
1.0+ | ✓ | 1.0+ | 1.3+ | 9.2+ |
Practice Your Knowledge
Quiz Time: Test Your Skills!
Ready to challenge what you've learned? Dive into our interactive quizzes for a deeper understanding and a fun way to reinforce your knowledge.