Here we look at properties that can assist in styling your text
- Text color
- Text alignment
- Text decoration
- Text transform
- Text shadow
- Text indentation
- Letter spacing
- Line height
- Word spacing
Text Color
The color property is used to set the text color. To specify the color you can use a color name (red), a HEX value (#ff0000) or an RGB value (rgb (255,0,0) ).
Example of the color property:.
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
</head>
<body>
<p style="color:#ff0000">This is some paragraph in red.</p>
</body>
</html>
Result
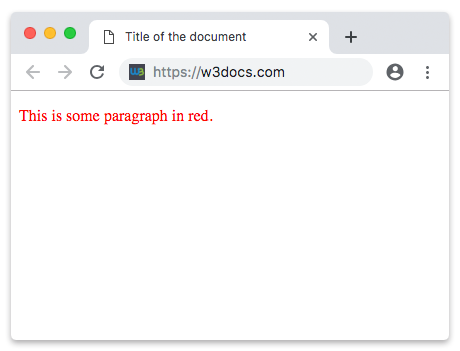
Text Alignment
Alignment property is used for aligning text inside an element left, right, center, etc.
Text alignment has four values:
- Left (text-align: left) - aligns the text to the left
- Right (text-align: right) - aligns the text to the right
- Center (text-align: center) - puts the text in center of the page
- Justify (text-align: justify) - stretches the line of text to align both the left and right ends (like in magazines and newspapers)
Browsers by default align text to the left, and if there is need to align text to the right or put it in the center, we should use the corresponding value.
Example of the text-alignment property:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
</head>
<body>
<p>This is some paragraph</p>
<p style="text-align:center">Some paragraph with value center.</p>
<p style="text-align:right">Some paragraph with value right.</p>
<p style="text-align:justify">Some paragraph with value justify.</p>
</body>
</html>
Text Decoration
Text decoration is used for setting the decoration of the text. In CSS3, it is a shorthand for the CSS text-decoration-line, CSS text-decoration-color and CSS text-decoration-style properties.
Decoration property is used to specify line decorations added to the text. The following values are valid for text-decoration property.
- Overline (text-decoration:overline) - each line of text has a line over it
- Underline (text-decoration:underline) - each line text is underlined
- Line-through (text-decoration:line-through) - each line of text has a line going through it
- None (text-decoration:none) - no text decoration is applied
Example of the text-decoration property:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
</head>
<body>
<a style="text-decoration:none">This is link without underline</a>
<h1 style="text-decoration:overline">Heading with value overline.</h1>
<p style="text-decoration:line-through">Some paragraph with value line-through.</p>
<a style="text-decoration:underline">Some hyperlink with value underline.</a>
</body>
</html>
Result
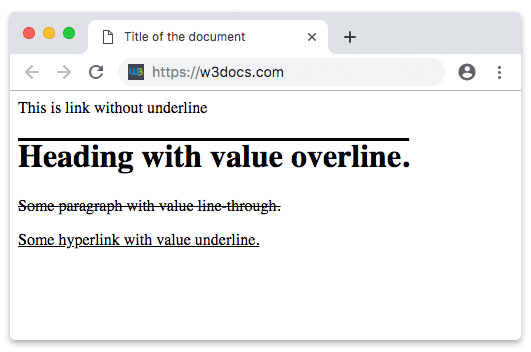
Text Transform
Transform property is used for controlling text capitalization. It means that you can set your text to be uppercase, lowercase, or capitalized (title case).
Transform property has the following values:
- Uppercase (text-transform: uppercase) - converts all characters to uppercase
- Lowercase (text-transform: lowercase) - converts all characters to lowercase
- Capitalize (text-transform: capitalize) - converts the first character of each word to uppercase
Example of the text-transform property:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
</head>
<body>
<p style="text-transform:uppercase">Paragraph with uppercase.</p>
<p style="text-transform:lowercase">Paragraph with lowercase.</p>
<p style="text-transform:capitalize">Paragraph with capitalize.</p>
</body>
</html>
Result
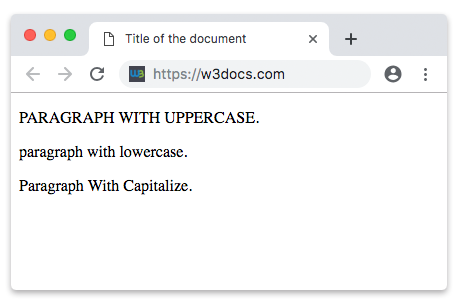
Text Shadow
We use the text-shadow property when we want to give shadow to our text.
Shadow property is used to apply various shadow effects to the text. It accepts a list of values. Each item in the list can have two and more comma-separated values.
The text shadow property syntax can look like
text-shadow: h-shadow v-shadow blur color
Here you can see examples of text shadow.
Text Indentation
Text indentation property is used for specifying the length of empty space of the first line in a text block. The values below are valid for this property:
- Length , which specifies the indentation in px, pt, cm, em, etc. The default value is 0. Negative values are allowed.
- Percentage - which Specifies the indentation in percentage of the width of the containing block.
- Each-line, when the indentation affects the first line as well as each line after a forced line break, but does not affect lines after a soft wrap break.
- Hanging, which inverts which lines are indented. The first line is not indented.
- Initial, which makes the property use its default value.
- Inherit, which inherits the property from its parent’s element.
Example of the text-indent property:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
p {
text-indent: 100px;
line-height: 24px;
font-size: 16px;
}
</style>
</head>
<body>
<h2>Text Indentation Example</h2>
<p>
Lorem ipsum, or lipsum as it is sometimes known, is dummy text used in laying out print, graphic or web designs. The passage is attributed to an unknown typesetter in the 15th century who is thought to have scrambled parts of Cicero's De Finibus Bonorum et Malorum for use in a type specimen book.
</p>
</body>
</html>
Letter Spacing
CSS letter-spacing property allows to define the spaces between letters/characters in a text. The following values are supported by this property:
- Normal, which means that there won’t be extra spaces between characters. It is the default value of this property.
- Length, which defines an extra space between characters. Negative values are allowed.
- Initial, which makes the property use its default value.
- Inherit, which inherits the property from its parent’s element.
Example of the letter-spacing property:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
p {
text-indent: 100px;
line-height: 24px;
font-size: 16px;
letter-spacing: 5px;
}
h3 {
letter-spacing: -1px;
}
</style>
</head>
<body>
<h2>Example of letter-spacing property</h2>
<p>
Lorem ipsum, or lipsum as it is sometimes known, is dummy text used in laying out print, graphic or web designs. The passage is attributed to an unknown typesetter in the 15th century who is thought to have scrambled parts of Cicero's De Finibus Bonorum et Malorum for use in a type specimen book.
</p>
<h3>
Here is some text with letter-spacing property.
</h3>
</body>
</html>
Line Height
The line-height property defines the line-height. It is used to set the leading of lines of a text. If the line-height value is greater than the value of the font-size of an element, the difference will be the leading of text. Here are the values supported by this property:
- Normal, which defines a normal line height. It is the default value of this property.
- Length, which defines a fixed line height in px, cm etc.
- Number, which defines a number which is multiplied with the current font size to set the line height.
- %, which defines a line height in percent of current font size.
- Initial, which makes the property use its default value.
- Inherit, which inherits the property from its parent’s element.
Example of the line-height property:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
p {
line-height: 30px;
}
h3 {
line-height: 1;
}
</style>
</head>
<body>
<h2>Example of line-height property</h2>
<p> Lorem ipsum, or lipsum as it is sometimes known, is dummy text used in laying out print, graphic or web designs. The passage is attributed to an unknown typesetter in the 15th century who is thought to have scrambled parts of Cicero's De Finibus Bonorum et Malorum for use in a type specimen book. </p>
<h3>
Lorem ipsum, or lipsum as it is sometimes known, is dummy text used in laying out print, graphic or web designs. The passage is attributed to an unknown typesetter in the 15th century who is thought to have scrambled parts of Cicero's De Finibus Bonorum et Malorum for use in a type specimen book.
</h3>
</body>
</html>
Word Spacing
With the help of the CSS word-spacing property we can change the space between the words in a piece of text, not the individual characters. It supports the values below:
- Normal, which specifies normal word spacing. This is the default value of this property.
- Length, which specifies an extra word spacing. Can be specified in px, pt, cm, em, etc. Negative values are valid.
- Initial, which makes the property use its default value.
- Inherit, which inherits the property from its parent’s element.
Example of the word-spacing property:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
p {
word-spacing: 1em;
}
h3 {
word-spacing: -3px;
}
span {
display: block;
word-spacing: 3rem;
}
</style>
</head>
<body>
<h2>Example of word-spacing property</h2>
<p>
Lorem ipsum, or lipsum as it is sometimes known, is dummy text used in laying out print, graphic or web designs. The passage is attributed to an unknown typesetter in the 15th century who is thought to have scrambled parts of Cicero's De Finibus Bonorum et Malorum for use in a type specimen book.
</p>
<h3>
Lorem ipsum, or lipsum as it is sometimes known, is dummy text used in laying out print, graphic or web designs. The passage is attributed to an unknown typesetter in the 15th century who is thought to have scrambled parts of Cicero's De Finibus Bonorum et Malorum for use in a type specimen book.
</h3>
<span>
Lorem ipsum, or lipsum as it is sometimes known, is dummy text used in laying out print, graphic or web designs.
</span>
</body>
</html>
Practice Your Knowledge
Quiz Time: Test Your Skills!
Ready to challenge what you've learned? Dive into our interactive quizzes for a deeper understanding and a fun way to reinforce your knowledge.