The <td> tag specifies a standard data cell in an HTML table. It must be used as a child element of <tr>, which defines a row in a table. To define a header cell the <th> tag is used.
The <td> tag can contain text, form, image, table etc. The content inside it is left-aligned by default.
All the rows in the table contain an equal number of cells, which is equivalent to the number of cells in the longest row. If there are less cells in a row, then the browser will automatically fill the row, placing empty cells at the end of it.
If you need to emphasize that there is no data in other cells, then create cell without content where it is necessary.
The cells added by browser have no borders, and if they go after each other, they will be shown as one integrated cell.
Syntax
The <td> tag comes in pairs. The content is written between the opening (<td>) and closing (</td>) tags.
<table>
<tr>
<td>...</td>
</tr>
</table>
Example of the HTML <td> tag:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
table {
width: 80%;
margin: 30px auto;
border-collapse: collapse;
}
tr {
background-color: lightgrey;
}
tr:first-child {
background-color: #1c87c9;
color: #fff;
}
th,
td {
padding: 10px;
border: 1px solid #666;
}
</style>
</head>
<body>
<table>
<tr>
<th>Month</th>
<th>Date</th>
</tr>
<tr>
<td>March</td>
<td>10.09.2018</td>
</tr>
<tr>
<td>June</td>
<td>18.07.2018</td>
</tr>
</table>
</body>
</html>
In this example we use <tr> tag to define table rows, <th> to define header cells, and <td> to define standard data cells.
The colspan and rowspan attributes are commonly used with the <td> tag to let the content span over multiple columns or rows.
Example of the HTML <td> tag with the colspan attribute:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
table {
width: 80%;
margin: 30px auto;
border-collapse: collapse;
}
tr {
background-color: #e6ebef;
}
tr:first-child {
background-color: #1c87c9;
color: #fff;
}
tr:last-child {
height: 60px;
}
tr:last-child td {
background-color: #a3cced;
}
tr:last-child span {
font-size: 14px;
}
th,
td {
padding: 10px;
border: 1px solid #666;
}
</style>
</head>
<body>
<table>
<tr>
<th>Company e-mail</th>
<th>Date</th>
</tr>
<tr>
<td>
<a href="#">[email protected]</a>
</td>
<td>01.09.2017</td>
</tr>
<tr style="height:60px;">
<td colspan="2" valign="bottom">[email protected];
<strong>01.09.2017 </strong>
<span>(received date)</span>
</td>
</tr>
</table>
</body>
</html>
Result
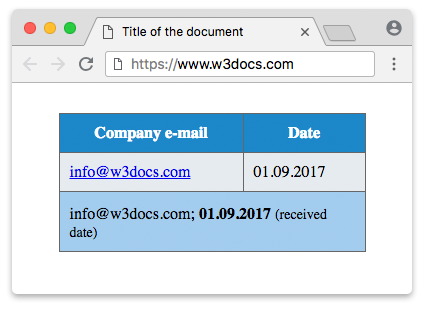
Example of the HTML <td> tag with the rowspan attribute:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
th, td {
padding: 10px;
border: 1px solid #666;
}
</style>
</head>
<body>
<table style="width:80%; margin:30px auto; border-collapse:collapse;">
<tr style="background-color:#1c87c9; color:#fff;">
<th>Month</th>
<th>Year</th>
</tr>
<tr>
<td style="background-color:#e6ebef;">March</td>
<td rowspan="2" style="background-color:#a3cced; text-align:center;">2014</td>
</tr>
<tr style="background-color:#e6ebef;">
<td style="background-color:#e6ebef;">April</td>
</tr>
</table>
</body>
</html>
<td> layout issues
By default, table data elements are laid out vertically aligned with the table data elements in previous and following rows. For example, if a table contains four rows of data cells, the first data cell from each row is aligned with the first data cell of other rows. And if you add an additional <td> element to a single row, the result will be unattractive. With the help of the colspan attribute you can solve this problem. Here, the cells that are located below and above the additional cell will be told to span throughout two table data cells.
Attributes
Attribute | Value | Description |
---|---|---|
abbr | text | Defines an abbreviated version of the content in a cell, or an alternative text. (User-agents, such as speech readers, may present this description before the content itself).
Not supported in HTML 5. |
align | left right center justify char |
Aligns the content in a cell.
Not supported in HTML 5. |
axis | category_name | Categorizes cells having similar content.
Not supported in HTML 5. |
background | background | Sets the background in a cell.
Not supported in HTML 5. |
bgcolor |
rgb(x,x,x)
#xxxxxx colorname |
Defines the background color of a cell.
Not supported in HTML 5. |
bordercolor | bordercolor | Sets the color of the border.
Not supported in HTML 5. |
char | character |
Aligns the content in a cell to a character. Is used only if attribute align="char".
Not supported in HTML 5. |
charoff | number |
Sets the number of characters the content will be aligned from the character specified by the char attribute.
Is used only if attribute align="char".
Not supported in HTML 5. |
colspan | number | Defines the number of columns a cell should span. The value of the attribute should be a positive integer. Default value is 1. |
headers | header_id | Specifies one or more header cells (defined by the <th> tag) a standard cell is related to. |
height | % pixels |
Sets the height of a cell.
Not supported in HTML 5. |
nowrap | nowrap | Specifies that the content inside a cell should not wrap. Not supported in HTML 5. |
rowspan | number | Specifies the number of rows a cell should span. The value of the attribute should be a positive integer. Default value is 1. It is not recommended to use values higher than 65534, as they will be clipped down to 65534. |
scope | col colgroup row rowgroup |
Defines the cells that the header (defined in the <th>) element relates to.
Not supported in HTML 5. |
valign |
top
middle bottom baseline |
Specifies vertical alignment of the content inside a cell.
Not supported in HTML 5. |
width | % pixels |
Sets the width of a cell.
Not supported in HTML 5. |
The <td> tag supports the Global Attributes and the Event Attributes.
How to style <td> tag?
Common properties to alter the visual weight/emphasis/size of text in <td> tag:
- CSS font-style property sets the style of the font. normal | italic | oblique | initial | inherit.
- CSS font-family property specifies a prioritized list of one or more font family names and/or generic family names for the selected element.
- CSS font-size property sets the size of the font.
- CSS font-weight property defines whether the font should be bold or thick.
- CSS text-transform property controls text case and capitalization.
- CSS text-decoration property specifies the decoration added to text, and is a shorthand property for text-decoration-line, text-decoration-color, text-decoration-style.
Coloring text in <td> tag:
- CSS color property describes the color of the text content and text decorations.
- CSS background-color property sets the background color of an element.
Text layout styles for <td> tag:
- CSS text-indent property specifies the indentation of the first line in a text block.
- CSS text-overflow property specifies how overflowed content that is not displayed should be signalled to the user.
- CSS white-space property specifies how white-space inside an element is handled.
- CSS word-break property specifies where the lines should be broken.
Other properties worth looking at for <td> tag:
- CSS text-shadow property adds shadow to text.
- CSS text-align-last property sets the alignment of the last line of the text.
- CSS line-height property specifies the height of a line.
- CSS letter-spacing property defines the spaces between letters/characters in a text.
- CSS word-spacing property sets the spacing between words.
Browser support
|
|
|
|
|
---|---|---|---|---|
✓ | ✓ | ✓ | ✓ | ✓ |
Practice Your Knowledge
Quiz Time: Test Your Skills!
Ready to challenge what you've learned? Dive into our interactive quizzes for a deeper understanding and a fun way to reinforce your knowledge.