The <progress> tag is one of the HTML5 elements. It is used to display the progress of the task (progress bar). Dynamically changing values of the progress bar must be defined with the scripts (JavaScript).
The appearance of the element can differ, depending on the browser and operating system.
We recommend using the <meter> tag to represent a gauge (e.g., the relevance of a query result).
Syntax
The <progress> tag comes in pairs. The content is written between the opening (<progress>) and closing (</progress>) tags.
Example of the HTML <progress> tag:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
</head>
<body>
<span>Loading:</span>
<progress value="35" max="100"></progress>
</body>
</html>
Result
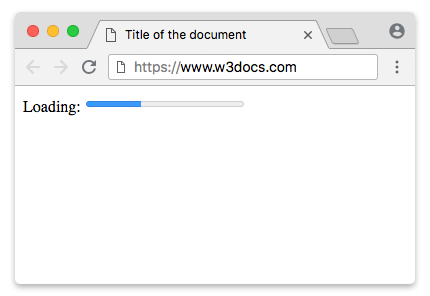
Progress bar
A progress bar can be indeterminate and determinate.
It's easier to style the indeterminate progress bar, as it doesn't have the value attribute. We can style it using the CSS negation clause :not().
The determinate progress bar is targeted by the progress[value] selector. Add dimensions to your progress bar with the CSS width and height properties and set the appearance to none:
Styling progress bars
WebKit/Blink (Chrome/Safari/Opera)
Chrome, Safari, and the latest version of Opera (16+) belong to this category. Styling the appearance of the </progress> element can be done with the use of -webkit-appearance: progress-bar.
Set -webkit-appearance: none; to reset the default styles.
progress[value] {
-webkit-appearance: none;
appearance: none;
width: 200px;
height: 15px;
}
Example of the determinate state of the progress bar:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
progress[value] {
-webkit-appearance: none;
appearance: none;
width: 200px;
height: 15px;
}
</style>
</head>
<body>
<span>Loading:</span>
<progress value="30" max="100"></progress>
</body>
</html>
After this, you may have problems because separate pseudo-classes are provided by different browsers for styling the progress bar. To solve this problem, you can use fallbacks.
WebKit/Blink provides two pseudo-classes:
- -webkit-progress-bar, which styles the progress element container.
- -webkit-progress-value, which styles the value inside the progress bar.
Style the -webkit-progress-bar with different CSS properties:
progress[value]::-webkit-progress-bar {
background-color: #eee;
border-radius: 2px;
box-shadow: 0 3px 6px rgba(0, 0, 0, 0.26) inset;
}
Style the -webkit-progress-value, which is the same as the bar, with several gradient backgrounds for different purposes. Use the -webkit- prefix for the gradients:
progress[value]::-webkit-progress-value {
background-image: -webkit-linear-gradient(-45deg, transparent 33%, rgba(0, 0, 0, .2) 33%, rgba(0, 0, 0, .2) 66%, transparent 66%), -webkit-linear-gradient(top, rgba(255, 255, 255, .25), rgba(0, 0, 0, .25)), -webkit-linear-gradient(left, #1000ff, #359900);
border-radius: 4px;
background-size: 20px 15px, 100% 100%, 100% 100%;
}
Example of the HTML <progress> tag used with CSS properties:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
progress[value] {
-webkit-appearance: none;
appearance: none;
width: 200px;
height: 15px;
}
progress[value]::-webkit-progress-bar {
background-color: #cccccc;
border-radius: 4px;
}
progress[value]::-webkit-progress-value {
background-image: -webkit-linear-gradient(-45deg, transparent 33%, rgba(0, 0, 0, .2) 33%, rgba(0, 0, 0, .2) 66%, transparent 66%), -webkit-linear-gradient(top, rgba(255, 255, 255, .25), rgba(0, 0, 0, .25)), -webkit-linear-gradient(left, #1000ff, #359900);
border-radius: 4px;
background-size: 20px 15px, 100% 100%, 100% 100%;
}
</style>
</head>
<body>
<span>Loading:</span>
<progress value="55" max="100"></progress>
</body>
</html>
Firefox
By using appearence: none we can remove the default bevel and emboss. However, this leaves a slight border in Firefox, which can be removed by using border: none. This solves the border issue with Opera 12 as well.
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
progress[value] {
-webkit-appearance: none;
-moz-appearance: none;
appearance: none;
border: none;
width: 200px;
height: 15px;
}
</style>
</head>
<body>
<span>Loading:</span>
<progress value="55" max="100"></progress>
</body>
</html>
Firefox provides a single pseudo-class (-moz-progress-bar) that can be used to target the progress bar value. In other words, we cannot style the background of the container in Firefox.
progress[value]::-moz-progress-bar {
background-image: -moz-linear-gradient( 135deg, transparent 33%, rgba(0, 0, 0, 0.1) 33%, rgba(0, 0, 0, 0.1) 66%, transparent 66%), -moz-linear-gradient( top, rgba(255, 255, 255, 0.25), rgba(0, 0, 0, 0.25)), -moz-linear-gradient( left, #ff00f7, #4e922a);
background-size: 35px 20px, 100% 100%, 100% 100%;
}
Firefox doesn't support the ::before or ::after pseudo-classes on the progress bar, and doesn't allow CSS3 keyframe animation on the progress bar, which provides a reduced experience.
Example of the HTML <progress> tag for Firefox:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
progress[value]::-moz-progress-bar {
background-image: -moz-linear-gradient( 135deg, transparent 33%, rgba(0, 0, 0, 0.1) 33%,
rgba(0, 0, 0, 0.1) 66%, transparent 66%),
-moz-linear-gradient( top, rgba(255, 255, 255, 0.25),
rgba(0, 0, 0, 0.25)),
-moz-linear-gradient( left, #ff00f7, #4e922a);
background-size: 35px 20px, 100% 100%, 100% 100%;
}
</style>
</head>
<body>
<span>Loading:</span>
<progress value="35" max="100"></progress>
</body>
</html>
Example of a cool progress bar use case by scrolling the page!
Here's an example of how to create a progress bar that shows how far you have scrolled down the page:
<!DOCTYPE html>
<html>
<head>
<style>
#progress-bar {
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 5px;
background-color: #ddd;
}
#progress-bar-fill {
height: 100%;
background-color: blue;
width: 0%;
}
</style>
</head>
<body>
<div id="progress-bar">
<div id="progress-bar-fill"></div>
</div>
<h1>Scrollable Content</h1>
<p>
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Sed auctor quam non felis interdum pellentesque. Suspendisse potenti. Nullam molestie neque in justo consectetur, sit amet varius arcu malesuada. Fusce sed laoreet ipsum. Nulla
facilisi. Donec eleifend auctor purus, eu bibendum risus facilisis sit amet.
</p>
<p>
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Sed auctor quam non felis interdum pellentesque. Suspendisse potenti. Nullam molestie neque in justo consectetur, sit amet varius arcu malesuada. Fusce sed laoreet ipsum. Nulla
facilisi. Donec eleifend auctor purus, eu bibendum risus facilisis sit amet.
</p>
<p>
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Sed auctor quam non felis interdum pellentesque. Suspendisse potenti. Nullam molestie neque in justo consectetur, sit amet varius arcu malesuada. Fusce sed laoreet ipsum. Nulla
facilisi. Donec eleifend auctor purus, eu bibendum risus facilisis sit amet.
</p>
<p>
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Sed auctor quam non felis interdum pellentesque. Suspendisse potenti. Nullam molestie neque in justo consectetur, sit amet varius arcu malesuada. Fusce sed laoreet ipsum. Nulla
facilisi. Donec eleifend auctor purus, eu bibendum risus facilisis sit amet.
</p>
<p>
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Sed auctor quam non felis interdum pellentesque. Suspendisse potenti. Nullam molestie neque in justo consectetur, sit amet varius arcu malesuada. Fusce sed laoreet ipsum. Nulla
facilisi. Donec eleifend auctor purus, eu bibendum risus facilisis sit amet.
</p>
<p>
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Sed auctor quam non felis interdum pellentesque. Suspendisse potenti. Nullam molestie neque in justo consectetur, sit amet varius arcu malesuada. Fusce sed laoreet ipsum. Nulla
facilisi. Donec eleifend auctor purus, eu bibendum risus facilisis sit amet.
</p>
<p>
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Sed auctor quam non felis interdum pellentesque. Suspendisse potenti. Nullam molestie neque in justo consectetur, sit amet varius arcu malesuada. Fusce sed laoreet ipsum. Nulla
facilisi. Donec eleifend auctor purus, eu bibendum risus facilisis sit amet.
</p>
<p>
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Sed auctor quam non felis interdum pellentesque. Suspendisse potenti. Nullam molestie neque in justo consectetur, sit amet varius arcu malesuada. Fusce sed laoreet ipsum. Nulla
facilisi. Donec eleifend auctor purus, eu bibendum risus facilisis sit amet.
</p>
<p>
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Sed auctor quam non felis interdum pellentesque. Suspendisse potenti. Nullam molestie neque in justo consectetur, sit amet varius arcu malesuada. Fusce sed laoreet ipsum. Nulla
facilisi. Donec eleifend auctor purus, eu bibendum risus facilisis sit amet.
</p>
<p>
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Sed auctor quam non felis interdum pellentesque. Suspendisse potenti. Nullam molestie neque in justo consectetur, sit amet varius arcu malesuada. Fusce sed laoreet ipsum. Nulla
facilisi. Donec eleifend auctor purus, eu bibendum risus facilisis sit amet.
</p>
<script>
window.addEventListener("scroll", function () {
var progressBarFill = document.getElementById("progress-bar-fill");
var scrollPosition = window.scrollY;
var totalHeight = document.body.scrollHeight - window.innerHeight;
var percentage = (scrollPosition / totalHeight) * 100;
progressBarFill.style.width = percentage + "%";
});
</script>
</body>
</html>
In this example, we have a fixed position div
element with an id
of progress-bar
that serves as the container for the progress bar. Inside this container, we have another div
element with an id
of progress-bar-fill
that serves as the actual progress bar.
We've used CSS to set the initial width and height of the progress bar, as well as the background colors for the progress bar and the progress bar fill.
We've also included a JavaScript event listener that listens for the scroll
event on the window object. When the user scrolls the page, we calculate the scroll position and the total height of the page, and then calculate the percentage of the page that has been scrolled. We update the width
property of the progress-bar-fill
element to reflect this percentage, thereby updating the progress bar.
You can copy this code into a new HTML file and open it in your web browser to see how it looks. As you scroll down the page, the progress bar will update to reflect how far you have scrolled. You can adjust the height and color of the progress bar to suit your needs.
Attributes
Attribute | Value | Description |
---|---|---|
max | number | Defines the maximum volume of the current process. The value can be a positive number bigger than the 0. |
value | number | Defines the size of the already completed task volume. The value can be a number from 0 to numbers, indicated in the max attribute, or a number in the range from 0 to 1, if the max attribute isn’t specified. |
The <progress> tag also supports the Global Attributes and the Event Attributes.
Browser support
|
|
|
|
---|---|---|---|
8+ | 16+ | 6+ | 11+ |
Practice Your Knowledge
Quiz Time: Test Your Skills!
Ready to challenge what you've learned? Dive into our interactive quizzes for a deeper understanding and a fun way to reinforce your knowledge.