A script is a small piece of a program embedded in web pages to make them dynamic and interactive. For example, with scripts, you can create a pop-up box message, a dropdown menu, etc. JavaScript is the most common scripting language used on websites. You can also use the <script> element with other languages (e.g. WebGL’s GLSl shading language).
How to Add Scripts
The <script> element is used to embed script or reference to an executable script within an HTML document. Scripts are usually nested within the <head> element to ensure that the script is ready to run when it is called. However, there is no restriction, and the script could be placed anywhere in the document.
If you need the same scripts to be available for many web pages, then you should place scripts into a separate file, then call it from the HTML document.
You can insert the following piece of code with the <script> tag into your HTML code:
<script type="text/javascript" src="scripts.js"></script>
How to Trigger Scripts
You can specify whether to make a script run automatically (as soon as the page loads) or after the user has done something (like hovering over or clicking a link). If you want the script to run if the user performs any action (called event), then you should use event handlers to let the browser know, which event is responsible for triggering individual script.
Event handlers are specified as attributes within the HTML tag.
Example of the HTML <script> tag with an event handler:
<!DOCTYPE html>
<html>
<head>
<title>Event Handler Example</title>
<script type="text/javascript">
function myAlert() {
alert("I am an event handler....");
return;
}
</script>
</head>
<body>
<span onmouseover="myAlert();">
Bring your mouse here to see an alert
</span>
</body>
</html>
The <noscript> element
Though many modern browsers support JavaScript, there are some older browsers that cannot run a JavaScript code, and also there's a probability that a user has disabled JavaScript on his/her browser. To provide alternative information for non-JavaScript browsers or browsers with JavaScript disabled, use the <noscript> tag. The contents of this tag are displayed to the user only in the case when JavaScript is disabled or when the browser doesn’t support JavaScript.
Example of the HTML <noscript> tag:
<!DOCTYPE html>
<html>
<head>
<title>Title of the documnet</title>
</head>
<body>
<script>
document.write("My first JavaScript example!")
</script>
<noscript>Sorry, your browser does not support JavaScript!</noscript>
<p>In case JavaScript is disabled or the browser doesn't support it, the code will display the content inside the noscript element.</p>
</body>
</html>
Result
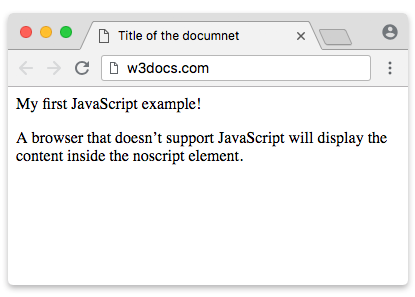
Practice Your Knowledge
Quiz Time: Test Your Skills!
Ready to challenge what you've learned? Dive into our interactive quizzes for a deeper understanding and a fun way to reinforce your knowledge.