The <canvas> tag is one of the HTML5 elements. It defines an area on the web page, where we can create different objects, images, animations, photo compositions via scripts (usually JavaScript). You should use a script to draw graphics because the <canvas> tag is just a container for graphics.
When working with canvas, it is important to distinguish between such concepts as the canvas element and the context of an element, that are often confused. This element is what's built into HTML (the DOM node). A canvas context is an object with its properties and method for rendering. Context can be 2D and 3D. The canvas element can have only one context.
An alternate content must be provided inside the <canvas> tag so that both the older browsers not supporting canvas and browsers with disabled JavaScript can render that content.
The exact size of a <canvas> element depends on the browser.
You can use CSS to change the displayed size of the canvas but it is better to specify the dimensions by setting the width and height attributes on <canvas>, either directly in HTML or by JavaScript.
Syntax
The <canvas> tag comes in pairs. The content is written between the opening (<canvas>) and closing (</canvas>) tags.
Example of the HTML <canvas> tag:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
</head>
<body>
<canvas id="canvasExample">Your browser doesn’t support the HTML5 canvas element.</canvas>
<script>
var c=document.getElementById('canvasExample');
var ctx=c.getContext('2d');
ctx.fillStyle='#1c87c9';
ctx.fillRect(10,50,80,80);
</script>
</body>
</html>
Result
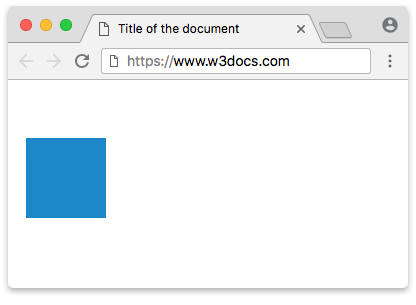
Example of the HTML <canvas> tag used for a text:
<!DOCTYPE HTML>
<html>
<head>
<title>Title of the document</title>
</head>
<body>
<canvas id="canvasExample" width="400" height="200"></canvas>
<script>
var canvas = document.getElementById('canvasExample');
var context = canvas.getContext('2d');
context.font = '30pt Calibri';
context.fillStyle = '#1c87c9';
context.fillText('Canvas Example !', 50, 100);
</script>
</body>
</html>
Example of the HTML <canvas> tag used for drawing a line:
<!DOCTYPE html>
<html>
<body>
<canvas width="300" height="150" style="border:1px solid #cccccc;" id="canvasExample">
Your browser does not support the HTML5 canvas tag.
</canvas>
<script>
var c = document.getElementById("canvasExample");
var ctx = c.getContext("2d");
ctx.moveTo(0,0);
ctx.lineTo(300,150);
ctx.strokeStyle='#1c87c9';
ctx.stroke();
</script>
</body>
</html>
Attributes
Attribute | Value | Description |
---|---|---|
height | pixels | Defines the element height in pixels. |
width | pixels | Defines the element width in pixels. |
The <canvas> tag supports Global Attributes and the Event Attributes.
Browser support
|
|
|
|
---|---|---|---|
4+ | 2+ | 3.1+ | 9+ |
Practice Your Knowledge
Quiz Time: Test Your Skills!
Ready to challenge what you've learned? Dive into our interactive quizzes for a deeper understanding and a fun way to reinforce your knowledge.