The <form> tag is used to add HTML forms to the web page for user input. Forms are used to pass the data submitted by the user to the server. The data is sent when pressing the "Submit" button. If there isn’t such button, the information is sent when the "Enter" key is pressed.
If the individual elements inside the <form> tag are valid you can use the CSS :valid pseudo class for styling the tag, and the :invalid pseudo class, in the case where they are not valid.
Syntax
The <form> tag comes in pairs. The content is written between the opening (<form>) and closing (</form>) tags.
The <form> element contains other HTML tags, which define the input method of data:
- The <input> tag defines a user input field.
- The <textarea> tag defines a form field to create a multiline input area.
- The <button> tag is used to place a button inside a form.
- The <select> tag sets up a control for creating a drop-down list box.
- The <option> tag defines the items in the drop-down list set by the <select> tag.
- The <optgroup> tag groups related data in the drop-down list set by the <select> tag.
- The <fieldset> tag visually groups the elements inside the form set by the <form> tag.
- The <label> tag sets the text label for the <input> element.
- The <legend> tag defines the header for the <fieldset> element.
Example of the HTML <form> tag:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
</head>
<body>
<form action="/form/submit" method="GET or POST" >
<label for="fname">Name</label>
<input type="text" name="FirstName" id="fname" value="Mary"/><br/><br/>
<label for="lname">Surname</label>
<input type="text" name="LastName"id="lname" value="Thomson"/><br/><br/>
<input type="submit" value="Submit"/>
</form>
</body>
</html>
Result
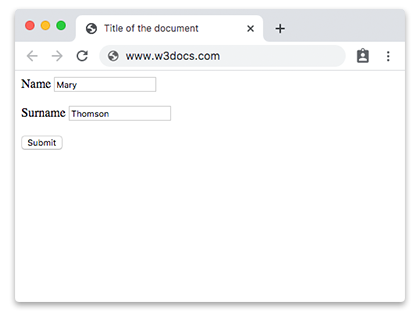
Example of the HTML <form> tag with the <input> and <label> tags:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
</head>
<body>
<form action="/form/submit" method="GET or POST" >
<label for="fname">Name</label>
<input type="text" name="Name" id="fname" value="Mary"/><br/><br/>
<label for="number">Phone</label>
<input type="number" name="Phone" id="number"/><br/><br/>
<label for="email">Email</label>
<input type="email" placeholder="Enter Email" name="email" required> <br/><br/>
<input type="submit" value="Submit"/>
</form>
</body>
</html>
Example of the HTML <form> tag with the <texarea> tag:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
</head>
<body>
<h1>Form example</h1>
<form action="/form/submit" method="GET or POST" >
<textarea rows="3" cols="30" placeholder="Type some text here"></textarea><br/>
<input type="submit" value="Submit"/>
</form>
</body>
</html>
Attributes
Attribute | Value | Description |
---|---|---|
accept | file_type | Specifies a comma-separated list of the types of files that the server accepts (the list can be presented via file uploads).
Not supported in HTML5. |
accept-charset | character_set | Specifies the encoding in which the server can receive and process data. |
action | URL | Indicates the address where the data is sent, and where it will be processed.While using this attribute change the "script name" to the name and location of the script file. |
autocomplete | on off |
Enables/disables auto-completion of form fields. The default value is on. |
enctype | application/x-www-form-urlencoded multipart/form-data text/plain | Determines how the form data is encoded as it is sent. (The default is application/x-www-form-urlencoded). (Used only with the POST method). |
method | get post |
Specifies the HTTP method for submitting form data. (the default method is GET). Sends form data in the address bar ("name = value"), which are added to the page URL after the question mark and are separated by an ampersand (&). (http://example.ru/doc/?name=Ivan&password=vanya)
|
name | text | Defines the form name. |
novalidate | novalidate | Sets that the data entered into the form will not be checked before being sent. |
target | Determines where to show the response received after submitting the form. | |
_blank | Shows in a new window. | |
_self | Shows in the current window. | |
_parent | Shows in the parent frame. | |
_top | Opens the entire width of the window. | |
framename | Opens in an iframe (the name must be specified as a value) |
The <form> tag also supports the Global attributes and the Event Attributes.
Browser support
|
|
|
|
|
---|---|---|---|---|
✓ | ✓ | ✓ | ✓ | ✓ |
Practice Your Knowledge
Which of the following methods are used to send form data in HTML?
Correct!
Incorrect!
Quiz Time: Test Your Skills!
Ready to challenge what you've learned? Dive into our interactive quizzes for a deeper understanding and a fun way to reinforce your knowledge.