How to Add Space Between Rows in the Table
Today’s task is to create space between two rows in a table. The space between two rows in a <table> can be added by using the CSS border-spacing and border-collapse properties. The border-spacing property is used to set the spaces between cells of a table, and the border-collapse property specifies whether the border of the table is collapsed or not. The border-spacing property can be used only when the border-collapse property is set to "separate".
Let’s see an example and show how to do that step by step.
Create HTML
- Place the <div> tag in the <body> section.
- Place the <h2> and <h3> tags and write some content in them.
- Place the <table> tag and create your table.
- Use the <tr> tag for each row.
- For the first row, use the <th> tag which defines a header cell in an HTML table.
- For the other rows, use the <td> tag which defines a standard data cell in an HTML table. For the cells belonging to the first row set a "td" class.
<body>
<div>
<h2>W3docs</h2>
<h3>Row spacing in a table</h3>
<table>
<tr>
<th>Employee ID</th>
<th>Name</th>
<th>Gender</th>
<th>Age</th>
</tr>
<tr>
<td class="td">10001</td>
<td>Tom</td>
<td>M</td>
<td>30</td>
</tr>
<tr>
<td class="td">10002</td>
<td>Sally</td>
<td>F</td>
<td>28</td>
</tr>
<tr>
<td class="td">10003</td>
<td>Emma</td>
<td>F</td>
<td>24</td>
</tr>
</table>
</div>
</body>
Add CSS
- Use the border-collapse property with its "separate" value for the table.
- Use the border-spacing property to set the distance between the borders of neighbouring table cells.
- For the first row, set the background color and the color of the text by using the background-color and color properties.
- Set the width and padding of the rows.
- Use the text-align property with the "center" value which aligns the text to the center.
- You can create a border around the cells of the table by using the border property and use the border-width, border-style and border-color properties.
- You can set the color of the <h2> element of the document by using the color property. Also, you can choose any color from our color picker.
table {
border-collapse: separate;
border-spacing: 0 15px;
}
th {
background-color: #4287f5;
color: white;
}
th,
td {
width: 150px;
text-align: center;
border: 1px solid black;
padding: 5px;
}
h2 {
color: #4287f5;
}
Here is the result of our code.
Example of adding space between horizontal rows:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
table {
border-collapse: separate;
border-spacing: 0 15px;
}
th {
background-color: #4287f5;
color: white;
}
th,
td {
width: 150px;
text-align: center;
border: 1px solid black;
padding: 5px;
}
h2 {
color: #4287f5;
}
</style>
</head>
<body>
<div>
<h2>W3docs</h2>
<h3>Row spacing in a table</h3>
<table>
<tr>
<th>Employee ID</th>
<th>Name</th>
<th>Gender</th>
<th>Age</th>
</tr>
<tr>
<td class="td">10001</td>
<td>Tom</td>
<td>M</td>
<td>30</td>
</tr>
<tr>
<td class="td">10002</td>
<td>Sally</td>
<td>F</td>
<td>28</td>
</tr>
<tr>
<td class="td">10003</td>
<td>Emma</td>
<td>F</td>
<td>24</td>
</tr>
</table>
</div>
</body>
</html>
Result
Employee ID | Name | Gender | Age |
---|---|---|---|
10001 | Tom | M | 30 |
10002 | Sally | F | 28 |
10003 | Emma | F | 24 |
Example of adding space between vertical columns:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
table {
border-collapse: separate;
border-spacing: 20px 0;
}
th {
background-color: #4287f5;
color: white;
}
th,
td {
width: 150px;
text-align: center;
border: 1px solid black;
padding: 5px;
}
h2 {
color: #4287f5;
}
</style>
</head>
<body>
<div>
<h2>W3docs</h2>
<h3>Row spacing in a table</h3>
<table>
<tr>
<th>Employee ID</th>
<th>Name</th>
<th>Gender</th>
<th>Age</th>
</tr>
<tr>
<td class="td">10001</td>
<td>Tom</td>
<td>M</td>
<td>30</td>
</tr>
<tr>
<td class="td">10002</td>
<td>Sally</td>
<td>F</td>
<td>28</td>
</tr>
<tr>
<td class="td">10003</td>
<td>Emma</td>
<td>F</td>
<td>24</td>
</tr>
</table>
</div>
</body>
</html>
In our first example, for the border-spacing property, we use a "0 15px" value which means that space is between the horizontal rows. In the second example, we use a "20px 0 " value which means that space is between the vertical rows.
A problem?!
Let's give some background to our table to see what we're talking about, so:
table {
border-collapse: separate;
border-spacing: 20px 0;
background: khaki; /* add this line */
}
And here's the result:
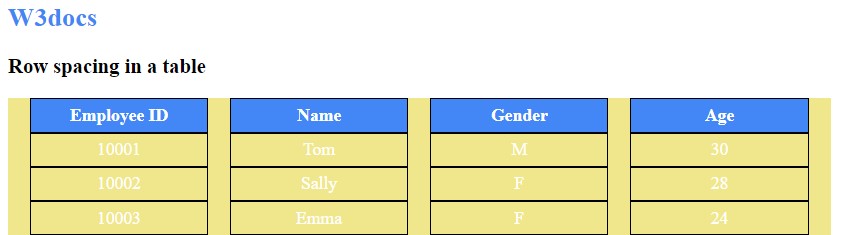
What if we want inner borders to be removed between the columns in this example? Now we have it in outer space of Employee ID and Age columns.
Ok, let's fix this together!
Remove the border-collapse: separate and border-spacing: 20px 0 from the table CSS.
Now, we will add the border-spacing: 20px 0 on each td of our table, instead of the whole table.
We should also add a display property of the block to have it work the way we want it to.
So, our changes would be like this:
table {
background: khaki;
}
table tbody {
display: block;
border-spacing: 20px 0;
}
The result will be the same as before. Now, its' time for us to delete the left and right outer border space. It can be done quickly by just adding the negative margin to the left and right of each td element so that it will remove that space for us.
table {
background: khaki;
}
table tbody {
margin: 0 -20px; /* add this line, -20px margin to left and right, 20px is based on the border-spacing amount which is 20 px in this example */
display: block;
border-spacing: 20px 0;
}
And here we go! This is precisely what we wanted! As you see, the left and right outer space have gone for good!
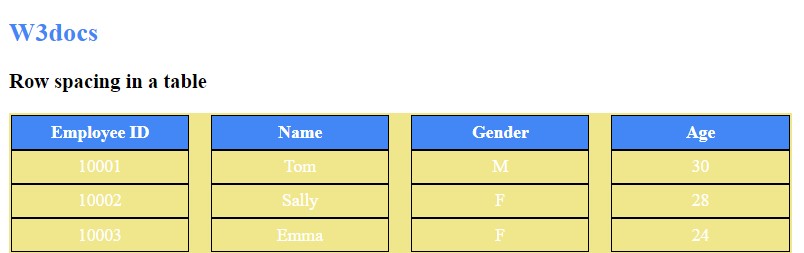
Now you can remove the background color as well and have your beautiful table!
Hope you enjoyed it, have a good time!