How to Add a Frame Around an Image
There are cases when building a web page you want to add a frame to the image without using Photoshop or any other editor. CSS can help you with this problem adding colorful frames with your preferred width and style to the image.
You can make a simple frame around an image by using the CSS border, padding and background properties.
Create HTML
- Create a <div> element with a class name "frame".
- Define an <img> tag in the <div> element.
- Set the alt attribute for the image.
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
</head>
<body>
<div class="frame">
<img src="/uploads/media/default/0001/01/b408569013c0bb32b2afb0f0d45e93e982347951.jpeg" alt="Nature">
</div>
</body>
</html>
Add CSS
- Set the height and width for the frame.
- Specify the style, the width and the color of the border with the border shorthand property.
- Set a background-color.
- Set the margin to "auto" and the padding with two values. The first value sets the top and bottom sides, and the second one sets the right and left sides.
- Set the width and height of the image to 100%.
.frame {
width: 300px;
height: 250px;
border: 3px solid #ccc;
background: #eee;
margin: auto;
padding: 15px 10px;
}
img {
width: 100%;
height: 100%;
}
Now, you can see the full example.
Example of adding a frame around an image:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
.frame {
width: 300px;
height: 250px;
border: 3px solid #ccc;
background: #eee;
margin: auto;
padding: 15px 10px;
}
img {
width: 100%;
height: 100%;
}
</style>
</head>
<body>
<div class="frame">
<img src="/uploads/media/default/0001/01/b408569013c0bb32b2afb0f0d45e93e982347951.jpeg" alt="Nature">
</div>
</body>
</html>
Result:
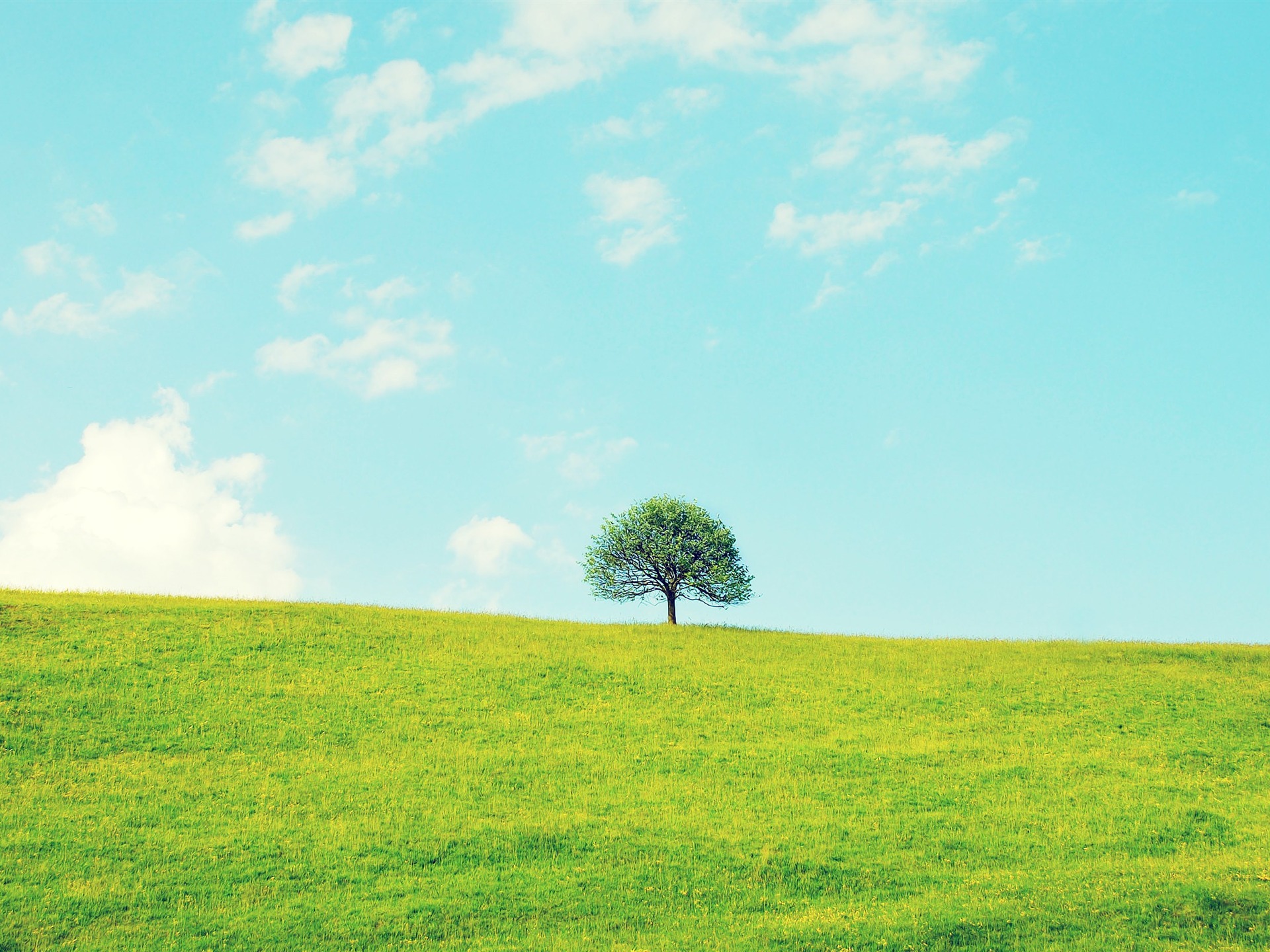
If you want to make a circle frame border for your image, you need to set the border-radius to 50% for all the sides of your border. Set the border-color, border-style, border-width properties according to your requirements. Do not forget to set the overflow property to "hidden" to make the rest of the image invisible.
Example of adding a circle frame around an image:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
.circle {
border-color: #666 #1c87c9;
border-image: none;
border-radius: 50% 50% 50% 50%;
border-style: solid;
border-width: 25px;
height: 200px;
width: 200px;
overflow: hidden;
}
img {
height: 100%;
width: 100%;
}
</style>
</head>
<body>
<div class="circle">
<img src="/uploads/media/default/0001/01/b408569013c0bb32b2afb0f0d45e93e982347951.jpeg" alt="Nature">
</div>
</body>
</html>
You can have different outputs by changing the border-radius and border-color properties. For example, if you want to have a square frame, you just need to set the border-radius to 0 for all the sides.
Example of adding a square frame around an image:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
.square {
height: 200px;
width: 200px;
border-color: #666 #1c87c9;
border-image: none;
border-radius: 0 0 0 0;
border-style: solid;
border-width: 30px;
}
img {
height: 100%;
width: 100%;
}
</style>
</head>
<body>
<div class="square">
<img src="/uploads/media/default/0001/01/b408569013c0bb32b2afb0f0d45e93e982347951.jpeg" alt="Nature">
</div>
</body>
</html>
If you want to have rounded corners for specific corners, set the border-radius to 50px for the corners you want to be rounded. In this case, also change the width and height according to your image size.
Example of adding a frame with rounded corners:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
.rounded-borders {
height: 200px;
width: 300px;
border-color: #666 #8ebf42;
border-image: none;
border-radius: 50px 0 50px 0;
border-style: solid;
border-width: 20px;
}
img {
height: 100%;
width: 100%;
}
</style>
</head>
<body>
<div class="rounded-borders">
<img src="/uploads/media/default/0001/01/b408569013c0bb32b2afb0f0d45e93e982347951.jpeg" alt="Nature">
</div>
</body>
</html>
How to Add a Border Image Frame to an Image
It is also possible to add an image as a border. For that purpose, CSS has a border-image property, which allows specifying an image as a border around an element.
You can define how to repeat the border-image in the following ways:
- stretch - the image is stretched to fill the area (this is a default value),
- repeat - the image is repeated to fill the area,
- round - the image is repeated to fill the area, (if it does not fill the area with a whole number of tiles, the image is rescaled, so it fits),
- space - the image is repeated to fill the area (if it does not fill the area with a whole number of tiles, the extra space is distributed around the tiles).
Example of adding a border-image frame:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
div {
width: 80%;
height: 300px;
margin-bottom: 20px;
background: url("/uploads/media/default/0001/01/b408569013c0bb32b2afb0f0d45e93e982347951.jpeg") no-repeat;
background-size: cover;
}
img {
width: 30%;
height: 30%;
}
.border-one {
border: 20px solid transparent;
border-image: url("/uploads/media/default/0001/01/812bf6a749522b8185c1beee20dd99dd6c6c87da.jpeg") 50 round;
}
.border-two {
border: 20px solid transparent;
border-image: url("/uploads/media/default/0001/01/812bf6a749522b8185c1beee20dd99dd6c6c87da.jpeg") 35% round;
}
.border-three {
border: 20px solid transparent;
border-image: url("/uploads/media/default/0001/01/812bf6a749522b8185c1beee20dd99dd6c6c87da.jpeg") 100% round;
}
.border-four {
border: 20px solid transparent;
border-image: url("/uploads/media/default/0001/01/812bf6a749522b8185c1beee20dd99dd6c6c87da.jpeg") 20 stretch;
}
</style>
</head>
<body>
<div class="border-one"></div>
<div class="border-two"></div>
<div class="border-three"></div>
<div class="border-four"></div>
<hr>
<p>Here is the original image:</p>
<img src="/uploads/media/default/0001/01/812bf6a749522b8185c1beee20dd99dd6c6c87da.jpeg" alt="Border">
</body>
</html>