Initially, JavaScript was created for web browsers. But, since then, it has expanded and become a language with different platforms and uses.
A platform can be a browser, a web-server, or another host. Each of them includes a platform-specific functionality. For JavaScript specification, it is a host environment. A host environment has its own objects and functions in addition to the language core. Web browsers allow controlling web pages. For instance, Node.js includes server-side features.
Let’s check out the overview of JavaScript, running in a web-browser:
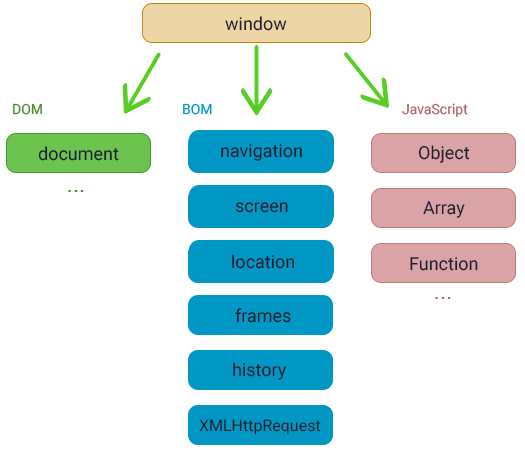
The “root” object named window has two roles that are the following:
- It is considered a global object for JavaScript code.
- It presents the “browser window”, providing methods to control it.
If you use it as a global object, it will look like this:
function welcome() {
console.log("Welcome to W3Docs");
}
// global functions are global object methods:
window.welcome();
You can also use it as a browser window. It is mainly performed for seeing the window height, like here:
Document Object Model (DOM)
Document Object Model (DOM) is targeted at representing the overall page content as objects that can be transformed.
The document object is considered the primary “entry point” to the page. It allows you to change or create anything on the page.
Below is a case of implementing a change using document.body.style:
The DOM specification explains a document structure, providing objects to manipulate it. Some non-browser instruments also use DOM.
For example, server-side scripts that download HTML pages and process them are also capable of using DOM. However, only a part of the specification is supported by them.
There exists a particular specification, CSS Object Model (CSSOM), which explains how they are represented as objects and the ways of reading and writing them.
CSSOM is used along with DOM whenever you modify style rules for the document. However, CSSOM is rarely used in practice, as CSS rules are considered static.
Browser Object Model (BOM)
The Browser Object Model (BOM) represents extra objects provided by the host environment to work with anything excluding the document.
For example, The navigator object provides background information about the operating system and the browser. There are various properties, but the most famous ones are navigator.userAgent (about the current browser) and navigator.platform (about the platform). The location object helps to read the current URL, and it can redirect your browser to a new one.
Here is the example of the location object use:
The functions such as alert/confirm/prompt are also a part of BOM. They are not related to the document directly, still representing pure browser methods of communicating with the user.
Please, note that BOM is a part of HTML specification.
As a conclusion, let’s state that JavaScript programs need a host environment. And, for a better understanding of client-side JavaScript, it is necessary to master the programming environment provided by a web browser.
Practice Your Knowledge
Quiz Time: Test Your Skills!
Ready to challenge what you've learned? Dive into our interactive quizzes for a deeper understanding and a fun way to reinforce your knowledge.