Introduction to JavaScript and the DOM
JavaScript, a powerful scripting language, plays a crucial role in modern web development. It enables dynamic interactions on web pages, making them not just informative but interactive and engaging. The Document Object Model (DOM), an essential aspect of web development, represents the structure of a webpage. It's a tree-like model that allows JavaScript to interact with HTML and XML documents, modifying content, structure, and styles dynamically.
Understanding the DOM
The DOM is a structured representation of your HTML document. It allows JavaScript to access and manipulate HTML elements, enabling real-time content changes, style adjustments, and interactive features. The DOM is organized as a tree, with each HTML element being a node. This hierarchical structure is key to understanding how JavaScript interacts with web pages.
Key Concepts:
- Nodes: Basic units of the DOM, representing HTML elements.
- Tree Structure: The hierarchical organization of nodes in the DOM.
- Document Object: The entry point to the DOM hierarchy.
Navigating the DOM Tree
JavaScript provides numerous methods to traverse the DOM tree, enabling developers to access and manipulate any element.
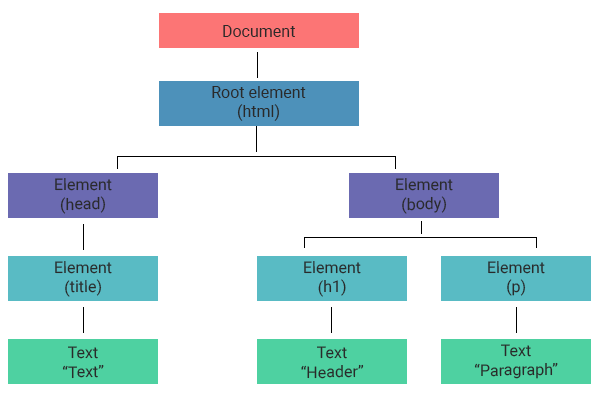
<!DOCTYPE html>
<html>
<head>
<title>Text</title>
</head>
<body>
<h1>Header</h1>
<p>Paragraph</p>
</body>
</html>
Core Methods:
-
document.getElementById()
: Accesses a single element by its ID. -
document.getElementsByClassName()
: Retrieves a list of elements with a specific class name. -
document.getElementsByTagName()
: Collects elements with a given tag name. -
document.querySelector()
: Returns the first element that matches a specified CSS selector. -
document.querySelectorAll()
: Returns a list of elements matching a CSS selector.
Manipulating DOM Elements
JavaScript's power lies in its ability to dynamically change the content and appearance of web pages.
Common Operations:
-
Changing Content: Use
element.innerHTML
orelement.textContent
. -
Modifying Styles: Access the
element.style
property. -
Creating Elements: Use
document.createElement()
. -
Adding Elements: Use
parentElement.appendChild(newElement)
. -
Removing Elements: Use
parentElement.removeChild(existingElement)
.
Event Handling in JavaScript
Events are actions or occurrences that happen in the system you are programming, which the system tells you about so you can respond to them in some way if desired. JavaScript can listen and respond to user actions like clicks, keyboard input, and page loading.
Event Listener Syntax:
element.addEventListener('event', functionToCall);
Key Events:
- Click Events: Triggered by user clicks.
- Load Events: Fired when resources are loaded.
- Input Events: Occur when users interact with input fields.
Event Handling Example
<!DOCTYPE html>
<html>
<head>
<title>Event Handling</title>
</head>
<body>
<button id="clickMe">Click Me</button>
<script>
document.getElementById('clickMe').addEventListener('click', function() {
alert('Button Clicked!');
});
</script>
</body>
</html>
Advanced DOM Manipulations
Beyond basic manipulations, JavaScript allows for more complex operations like cloning nodes, handling forms, and manipulating CSS classes.
Techniques:
- Cloning Nodes:
element.cloneNode(true)
. - Form Handling: Access and manipulate form elements.
- CSS classes Manipulation: Use
element.classList.add()
,element.classList.remove()
, orelement.classList.toggle()
.
JavaScript and DOM: Best Practices
To efficiently use JavaScript with the DOM, consider the following best practices:
- Minimize DOM Manipulations: Reduces re-rendering and improves performance.
- Use Event Delegation: Manages event listeners efficiently.
- Understand Reflow and Repaint: Optimize for performance.
JavaScript and DOM: Code Examples
Changing Content
<!DOCTYPE html>
<html>
<head>
<title>Sample Page</title>
</head>
<body>
<div id="content">Original Content</div>
<script>
document.getElementById('content').innerHTML = 'Updated Content';
</script>
</body>
</html>
Adding New Elements
<!DOCTYPE html>
<html>
<head>
<title>Add Elements</title>
</head>
<body>
<div id="container"></div>
<script>
var newElement = document.createElement('p');
newElement.textContent = 'New Paragraph';
document.getElementById('container').appendChild(newElement);
</script>
</body>
</html>
Remember, mastering JavaScript and the DOM is a continuous learning journey. Keep experimenting, building, and refining your skills to become a proficient JavaScript developer.
Practice Your Knowledge
Quiz Time: Test Your Skills!
Ready to challenge what you've learned? Dive into our interactive quizzes for a deeper understanding and a fun way to reinforce your knowledge.