How to Give a Text or Image a Transparent Background Using CSS
There is no property the same as transparency in CSS. However, you can create a transparency effect by using the CSS3 opacity property.
The opacity property specifies the image or text transparency. The number ranges between 0 and 1. The value of 1 is the default value and makes an element fully opaque. A value of 0 makes an element fully transparent. A value between 0 and 1 gradually makes an element clear.
Creating a Transparent Image
To create a simple transparent image, you need to set the opacity for your <img> element.
Example of creating a transparent image using the opacity property:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
.trans {
-moz-opacity: 0.5;
-khtml-opacity: 0.5;
opacity: 0.5;
}
</style>
</head>
<body>
<h2>Opacity: 0.5</h2>
<img class="trans" src="/uploads/media/default/0001/01/b5edc1bad4dc8c20291c8394527cb2c5b43ee13c.jpeg" alt="Image" width="200">
<h2>Original image</h2>
<img src="/uploads/media/default/0001/01/b5edc1bad4dc8c20291c8394527cb2c5b43ee13c.jpeg" alt="Original Image" width="200">
</body>
</html>
Result
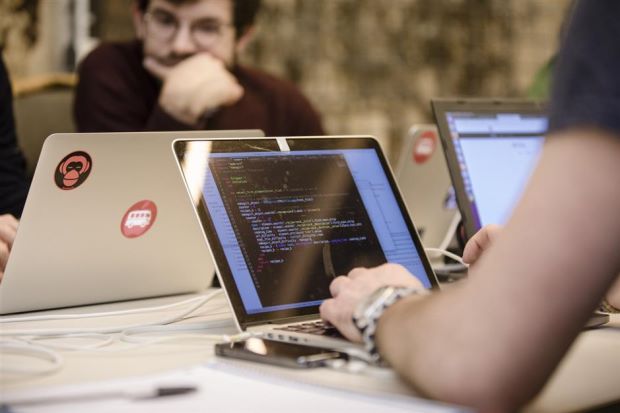
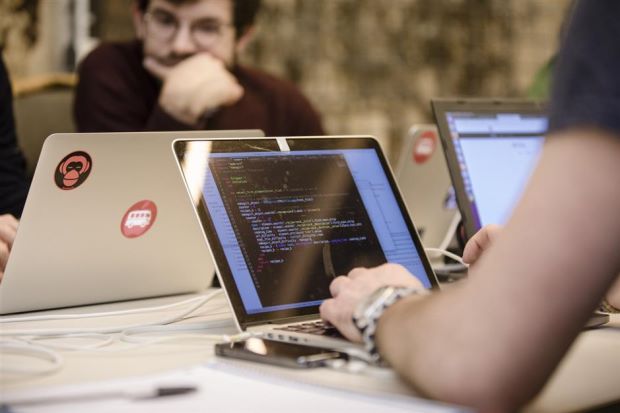
Here see an example where the image on the background is transparent due to the opacity: 0.5.
Example of creating a transparent background image:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
body {
background: url("/uploads/media/default/0001/01/b5edc1bad4dc8c20291c8394527cb2c5b43ee13c.jpeg") no-repeat;
background-size: cover;
}
.logo {
-moz-opacity: 0.5;
-khtml-opacity: 0.5;
opacity: 0.5;
}
div {
padding-top: 30px;
text-align: center;
}
</style>
</head>
<body>
<div>
<img class="logo" src="/build/images/w3docs-logo-black.png" alt="W3docs logo">
</div>
</body>
</html>
Now, let’s see an example where a transparent box, with a text inside, is created on a background image. Create a box using the <div> tag and add the padding-top and text-align properties to put the box in the middle of your background. Then, set the opacity for your box.
Example of creating a background image with a transparent box of text:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
body {
background: url("/uploads/media/default/0001/01/b5edc1bad4dc8c20291c8394527cb2c5b43ee13c.jpeg") no-repeat;
background-size: cover;
}
.logo {
-moz-opacity: 0.5;
-khtml-opacity: 0.5;
opacity: 0.5;
}
div {
padding-top: 30px;
text-align: center;
}
</style>
</head>
<body>
<div>
<img class="logo" src="/build/images/w3docs-logo-black.png" alt="Background Image">
</div>
</body>
</html>
In our next example, we use the :after pseudo-element, which is added to the "box" class.
Example of setting opacity with the :after selector:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
.box:after {
content: "";
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
background-image: linear-gradient(120deg, #eaee44, #33d0ff);
background-color: #666666;
opacity: 0.6;
-moz-opacity: 0.6;
-khtml-opacity: 0.6;
}
.box {
position: relative;
min-height: 50vh;
background: url("/uploads/media/default/0001/01/b5edc1bad4dc8c20291c8394527cb2c5b43ee13c.jpeg") no-repeat center top;
display: flex;
background-size: cover;
}
.box p {
margin: auto;
font-size: 30px;
z-index: 1;
position: relative;
}
</style>
</head>
<body>
<div class="box">
<p>This is a some text.</p>
</div>
</body>
</html>
Now, let's consider another case when the opacity effect disappears while hovering. Here, the opacity property is used with the :hover selector to change the opacity on mouse-over.
Example of setting a transparent background with a hovering effect:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
img {
-moz-opacity: 0.5;
-khtml-opacity: 0.5;
opacity: 0.5;
}
img:hover {
-moz-opacity: 1;
-khtml-opacity: 1;
opacity: 1;
}
</style>
</head>
<body>
<h2>Hover over the image to see the effect</h2>
<img src="/uploads/media/default/0001/01/4982c4f43023330a662b9baed5a407e391ae6161.jpeg" alt="House" width="300">
</body>
</html>