Lorem Ipsum is simply dummy text of the printing and typesetting industry.
How to Create an Image with Transparent Text with CSS
In this snippet, learn about the methods used to overlay text on images. For an ideal combination, the image should be dark, and the text light.
How to create an image with transparent text
This is not so difficult as it seems. It is quite simple, and you can achieve your desired result by using only CSS.
For having a simple, transparent text on an image, you need to have your image or background image in a <div> element, and the content block in another <div>.
Then, use the CSS opacity property to set a transparent image or text. The opacity property is used to adjust the transparency of a text or photo. The value of opacity can be between 0.0 to 1.0, where the low value demonstrates high transparency and the high value shows low transparency. The amount of the opacity is counted as Opacity% = Opacity * 100.
Or instead of the opacity property, you can just set an RGBA color value. See an example in action.
Example of creating an image with transparent text:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
* {
box-sizing: border-box;
}
.parent {
position: relative;
max-width: 800px;
margin: 0 auto;
}
.parent img {
vertical-align: middle;
}
.parent .text {
position: absolute;
bottom: 0;
background: rgb(0, 0, 0);/* Fallback color */
background: rgba(0, 0, 0, 0.5);/* Black background with opacity */
color: #ffffff;
width: 100%;
padding: 20px;
}
</style>
</head>
<body>
<h2>Transparent Text on an Image</h2>
<div class="parent">
<img src="https://www.w3docs.com/uploads/media/default/0001/03/faa2b10a44e1d88ddafbf7ab6002ce24659529d4.jpeg" alt="Notebook" style="width:100%;">
<div class="text">
<h2>Title</h2>
<p>
Lorem Ipsum is simply dummy text of the printing and typesetting industry. Lorem Ipsum has been the industry's standard dummy text ever since the 1500s, when an unknown printer took a galley of type and scrambled it to make a type specimen book.
</p>
</div>
</div>
</body>
</html>
Result
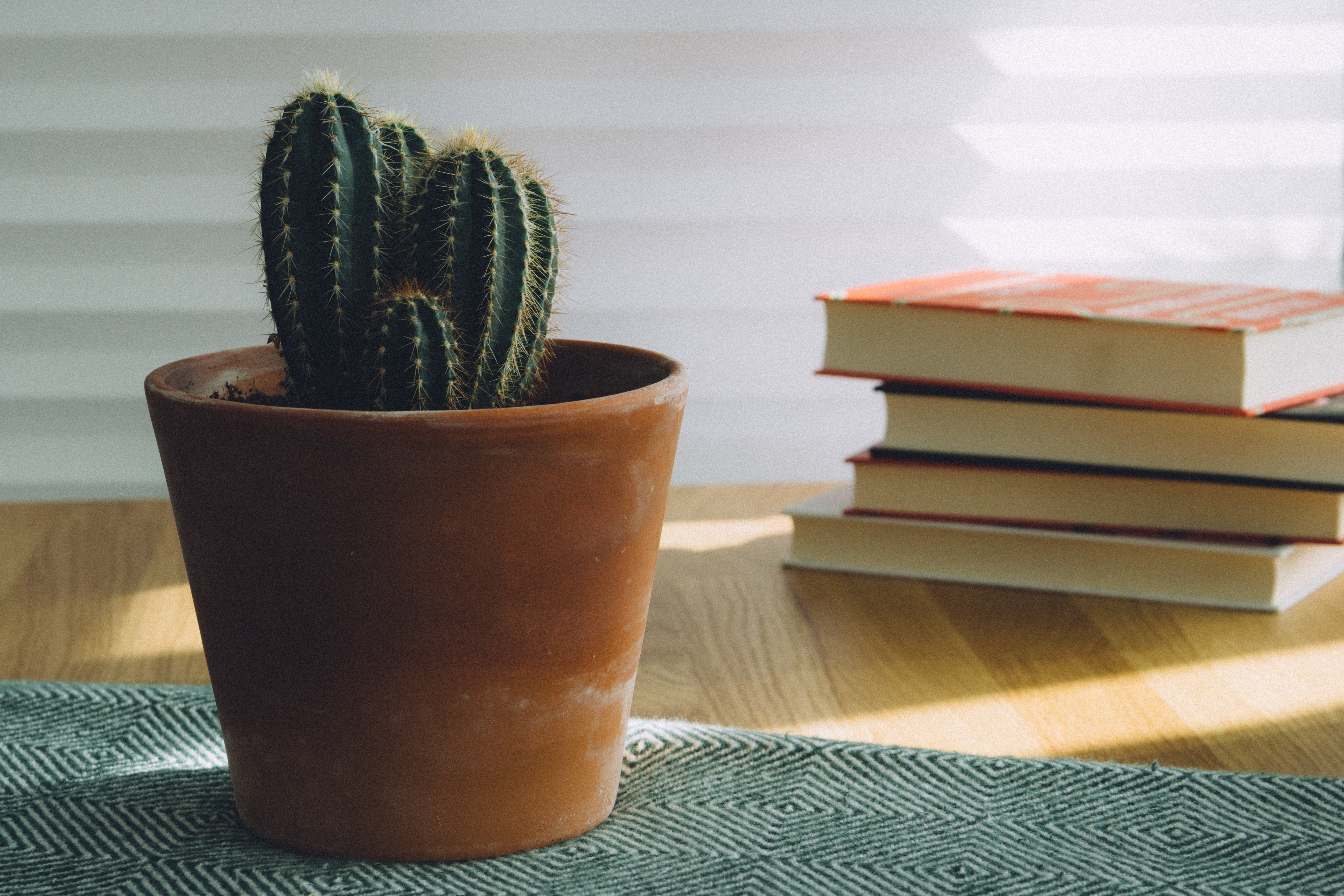
Let’s see another example where the text is set on a background image which fills the entire screen.
Example of adding a text on the background image:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
body {
font-family: 'Source Sans Pro', sans-serif;
}
header {
background: linear-gradient( rgba(0, 0, 0, 0.5), rgba(0, 0, 0, 0.5)), url(https://w3docs.com/uploads/media/default/0001/01/e4ecd8f48ea6eac1f966ccb81cd388241d54c948.jpeg);
background-size: cover;
height: 100vh;
}
.container {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
color: white;
text-align: center;
}
h1 {
text-transform: uppercase;
margin: 0;
font-size: 3rem;
white-space: nowrap;
}
</style>
</head>
<body>
<header>
<div class="container">
<h1>Cakes and Fruits</h1>
</div>
</header>
</body>
</html>
How to position a text over an image
To position the text over an image and define its place on the image, you need to apply the CSS position property set to its "absolute" value for the text blocks and the "relative" value for the parent element. Then, use the CSS top, bottom left, and right properties to define the position of an element in combination with the position property. And for centering the text block, apply the transform property like this: transform: translate(-50%, -50%).
Example of positioning the text over an image:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
.container {
position: relative;
text-align: center;
color: #000000;
font-size: 30px;
}
.bottom-left {
position: absolute;
bottom: 8px;
left: 16px;
}
.top-left {
position: absolute;
top: 8px;
left: 16px;
}
.top-right {
position: absolute;
top: 8px;
right: 16px;
}
.bottom-right {
position: absolute;
bottom: 8px;
right: 16px;
}
.centered {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
}
</style>
</head>
<body>
<h2>Positionet Text over an Image</h2>
<div class="container">
<img src="https://www.w3docs.com/uploads/media/default/0001/03/faa2b10a44e1d88ddafbf7ab6002ce24659529d4.jpeg" alt="Snow" style="width:100%;">
<div class="bottom-left">Bottom Left</div>
<div class="top-left">Top Left</div>
<div class="top-right">Top Right</div>
<div class="bottom-right">Bottom Right</div>
<div class="centered">Center</div>
</div>
</body>
</html>
How to add text blocks over an image
Here, let’s see how to create text in a box over images. In a reliable and straightforward way, a transparent text block can be added to an image. Whip up on some white text a slightly transparent black rectangle. If the overlay is sufficiently opaque, you can have almost any image below, and the text will still be completely readable.
As we have already learned how to make and position text blocks over an image above, now we need to use the CSS background property to add a background style and color to our text block.
Example of adding a dark text block over an image:
<!DOCTYPE html>
<html>
<head>
<title>Image with text block</title>
<style>
.parent {
position: relative;
}
.text-block {
position: absolute;
bottom: 50px;
left: 20px;
background-color: #000;
opacity: .6;
color: white;
padding-left: 20px;
padding-right: 20px;
}
.parent img {
width: 100%;
}
</style>
</head>
<body>
<h2>Text Block on an Image</h2>
<div class="parent">
<img src="https://www.w3docs.com/uploads/media/default/0001/03/faa2b10a44e1d88ddafbf7ab6002ce24659529d4.jpeg" alt="Books" />
<div class="text-block">
<h3>Books</h3>
<p>Do you like reading books?</p>
</div>
</div>
</body>
</html>
Here, see another example with dark and light text blocks.
Example of adding dark and light text blocks over an image:
<!DOCTYPE html>
<html>
<head>
<title>Image with text block</title>
<style>
.block {
margin: 10px;
width: 230px;
height: 150px;
float: left;
background: url(https://w3docs.com/uploads/media/default/0001/03/b847dafa71d0dc9d81db3ddda418e12934a5aa88.png);
background-size: cover;
position: relative;
}
h2 {
position: absolute;
bottom: 10px;
left: 10px;
background: rgba(0, 0, 0, 0.75);
padding: 4px 8px;
color: white;
margin: 0;
font: 14px Sans-Serif;
}
.light h2 {
background: rgba(255, 255, 255, 0.75);
color: black;
}
</style>
</head>
<body>
<div class="block">
<h2>Flying Birds</h2>
</div>
<div class="block light">
<h2>Flying Birds</h2>
</div>
</body>
</html>
How to add blur effect to the text block
The blurring part of the underlying image is an amazingly great way to make an overlaid text easy to read.
For having a blur effect, you need to set the filter property with the "blur" value (filter: blur) for your text block.
Example of adding a blur effect (4px) to the text block:
<!DOCTYPE html>
<html>
<head>
<title>Blur Effect on Text Block</title>
<style>
* {
box-sizing: border-box;
}
.text-block {
background: url(https://w3docs.com/uploads/media/default/0001/03/0a71a511b3381286ced8e2fc7f331296cd96649f.png);
background-attachment: fixed;
width: 300px;
height: 200px;
position: relative;
overflow: hidden;
margin: 20px;
}
.text-block > header {
position: absolute;
bottom: 0;
left: 0;
width: 100%;
padding: 20px 10px;
background: inherit;
background-attachment: fixed;
overflow: hidden;
}
.text-block > header::before {
content: "";
position: absolute;
top: -20px;
left: 0;
width: 200%;
height: 200%;
background: inherit;
background-attachment: fixed;
-webkit-filter: blur(4px);
filter: blur(4px);
}
.text-block > header::after {
content: "";
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
background: rgba(0, 0, 0, 0.25)
}
.text-block > header > h2 {
margin: 0;
color: white;
position: relative;
z-index: 1;
}
</style>
</head>
<body>
<h1>Blur Effect on Text Block</h1>
<div class="text-block">
<header>
<h2>
Blur Effect
</h2>
</header>
</div>
</body>
</html>
You can add the blur effect more to make your text easier to read.
Example of adding a blur effect (9px) to the text block:
<!DOCTYPE html>
<html>
<head>
<title>Blur Effect on Text Block</title>
<style>
* {
box-sizing: border-box;
}
.text-block {
background: url(https://w3docs.com/uploads/media/default/0001/03/0a71a511b3381286ced8e2fc7f331296cd96649f.png);
background-attachment: fixed;
width: 300px;
height: 200px;
position: relative;
overflow: hidden;
margin: 20px;
}
.text-block > header {
position: absolute;
bottom: 0;
left: 0;
width: 100%;
padding: 20px 10px;
background: inherit;
background-attachment: fixed;
}
.text-block > header::before {
content: "";
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
background: inherit;
background-attachment: fixed;
-webkit-filter: blur(9px);
filter: blur(9px);
transform: scale(2) translateY(20px);
}
.text-block > header > h2 {
margin: 0;
color: white;
position: relative;
z-index: 1;
}
</style>
</head>
<body>
<h1>Blur Effect on Text Block</h1>
<div class="text-block">
<header>
<h2>
Blur Effect
</h2>
</header>
</div>
</body>
</html>