How to Create a Multicolor Text with HTML and CSS
Multicolor texts can make your website more attractive and interesting for visitors. This snippet will help you to learn how to create such texts for your website.
There are 3 ways of creating a multicolor text. Let’s dive in and try it together.
Use image editors
You can create a multicolor text with the help of image editors such as Pixelmator, Photoshop and so on. So, you can create a multicolor image and embed it into your website.
As a result, your code will look like the following:
<img src="multicolortext.png" alt="multi color text"/>
Make separate elements
Another way is creating a text with separate elements by using the HTML <span> tag, which is an empty container. This means that you create a multicolor text by putting each letter in a separate element to define a different color for each letter of your text.
Example of creating a multicolor text with separate elements in HTML:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
</head>
<body>
<span style="color:#FF0000">H</span>
<span style="color:#66CC66">e</span>
<span style="color:#FF9966">l</span>
<span style="color:#FFCCCC">l</span>
<span style="color:#FF0066">o</span>
</body>
</html>
Result
Use CSS
And finally, you can use CSS. This is the easiest way of creating such kind of text.
Put your text in a <span> tag and give it a class name "multicolortext". Then, you need the CSS background-image property to add a gradient background to your text with its "linear-gradient" value, where you put the names of your preferred colors.
To get multicolor text instead of the multicolor background, you need to use the background-clip property, which lets you control how far a background image or color extends beyond the element's padding or content. This step gives some colors to your text, but not exactly what you want.
You can get the final result by making your text color transparent with the color property so that the background color will be reflected in the text.
Example of creating a multicolor text with HTML and CSS:
<!DOCTYPE html>
<html>
<head>
<title>The title of the document</title>
<style>
.multicolortext {
background-image: linear-gradient(to left, violet, indigo, green, blue, yellow, orange, red);
-webkit-background-clip: text;
-moz-background-clip: text;
background-clip: text;
color: transparent;
}
</style>
</head>
<body>
<h1>
<span class="multicolortext">Let’s be creative!</span>
</h1>
</body>
</html>
Let's get modern!
I think you're like me in that previous examples are not something we want to do in this day and age (Although they were good enough to grasp ways to do it).
So let's do it in a more modern way. Here comes the magic of linear-gradient! Now we start our example, going step by step, and extend this!
We have just one h1 tag and will make it alive soon with an animation!
Also, you can read the comments in front of each closing line of CSS styles to help you understand even better.
We start implementing our text with some basic styling.
<!DOCTYPE html>
<html lang="en">
<head>
<title>Linear Gradient</title>
</head>
<body>
<style>
h1 {
font-size: 10vw; /* make our h1 tag larger */
font-family: sans-serif; /* choosing our font */
}
</style>
<h1>W3DOCS</h1>
</body>
</html>
Here we'll add background and our linear-gradient value.
h1 {
font-size: 10vw;
/* make our h1 tag larger */
font-family: sans-serif;
/* choosing our font */
background: linear-gradient(to right, rgba(255, 215, 255, 0) 0%, rgba(225, 255, 255, 0.5) 20%, rgba(255, 255, 255, 0) 61%), linear-gradient(rgb(97, 183, 217) 52%, rgb(224, 246, 255) 60%, rgb(78, 99, 132) 61%);
/* you can change the colors based on your preference */
}
Let's see what we've got now:

Now, let's add background-clip and -webkit-text-fill-color properties to have the linear-gradient take effect on our text.
h1 {
font-size: 10vw;
/* make our h1 tag larger */
font-family: sans-serif;
/* choosing our font */
background: linear-gradient(to right, rgba(255, 215, 255, 0) 0%, rgba(225, 255, 255, 0.5) 20%, rgba(255, 255, 255, 0) 61%), linear-gradient(rgb(97, 183, 217) 52%, rgb(224, 246, 255) 60%, rgb(78, 99, 132) 61%);
/* you can change the colors based on your preference */
background-clip: text;
/*add this line, it defines how far the background should extend within an element, here we set it to text */
-webkit-background-clip: text;
/* add this line, for browsers compatibility */
-webkit-text-fill-color: transparent;
/* specifies the fill color of text characters. We use transparent to use the background value as our text fill */
}
Now it looks like this:
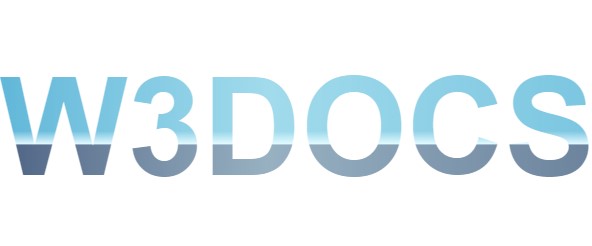
Much better. Let's take it a step further and give it some wavy animation to make it even cooler!
h1 {
font-size: 10vw;
/* make our h1 tag larger */
font-family: sans-serif;
/* choosing our font */
background: linear-gradient(to right, rgba(255, 215, 255, 0) 0%, rgba(225, 255, 255, 0.5) 20%, rgba(255, 255, 255, 0) 61%), linear-gradient(rgb(97, 183, 217) 52%, rgb(224, 246, 255) 60%, rgb(78, 99, 132) 61%);
/* you can change the colors based on your preference */
background-clip: text;
/*it defines how far the background should extend within an element, here we set it to text */
-webkit-background-clip: text;
/*for browsers compatibility */
-webkit-text-fill-color: transparent;
/* specifies the fill color of text characters. We use transparent to use the background as our text fill */
animation: wave 2000ms ease alternate infinite;
/* add this line */
transition: all 0.4s ease;
/* add this line */
}
@keyframes wave {
0% {
background-position: 0 0;
}
100% {
background-position: 50vw 10px;
}
}
/* add this line */
We defined a custom animation named wave here, which will be run every 2 seconds, and during this time, it will change our background-position 50vw on the x-axis and 10px on the y-axis. You're free to change that values and get your desired result as well!
We also declared a transition to smooth this movement a little bit!
Here's the final code and result:
<!DOCTYPE html>
<html lang="en">
<head>
<title>Linear Gradient</title>
</head>
<body>
<style>
h1 {
font-size: 10vw; /* make our h1 tag larger */
font-family: sans-serif; /* choosing our font */
background: linear-gradient(to right, rgba(255, 215, 255, 0) 0%, rgba(225, 255, 255, 0.5) 20%, rgba(255, 255, 255, 0) 61%), linear-gradient(rgb(97, 183, 217) 52%, rgb(224, 246, 255) 60%, rgb(78, 99, 132) 61%); /* you can change the colors based on your preference */
background-clip: text; /*it defines how far the background should extend within an element, here we set it to text */
-webkit-background-clip: text; /*for browsers compatibility */
-webkit-text-fill-color: transparent; /* specifies the fill color of text characters. We use transparent to use the background as our text fill */
animation: wave 2000ms ease alternate infinite;
transition: all 0.4s ease;
}
@keyframes wave {
0% {
background-position: 0 0;
}
100% {
background-position: 50vw 10px;
}
}
</style>
<h1>W3DOCS</h1>
</body>
</html>
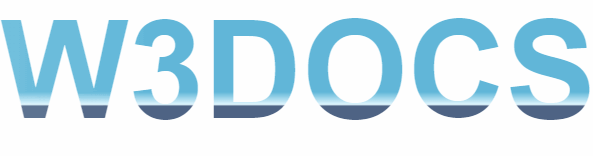
Cool! I hope you've enjoyed it!