How to Resize Images Proportionally for Responsive Web Design With CSS
One of the main parts of responsive web design is resizing the image automatically to fit the width of its container. Since nowadays people use different kinds of desktops, our web pages must be appropriate for all types of screens.
So, let’s learn three ways of resizing images and making responsive images with only HTML and CSS.
Resize images with the HTML width and height attributes
Apply the width and height attributes for your <img> tag to define the width and height of the image. See an example below.
Example of resizing an image proportionally with the width and height attributes:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
</head>
<body>
<p>Resized image:</p>
<img src="/uploads/media/default/0001/01/25acddb3da54207bc6beb5838f65f022feaa81d7.jpeg" alt="Aleq" width="160" height="145" />
<p>Original image size:</p>
<img src="/uploads/media/default/0001/01/25acddb3da54207bc6beb5838f65f022feaa81d7.jpeg" alt="Aleq" />
</body>
</html>
Result
Resized image:
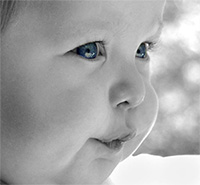
Original image size:
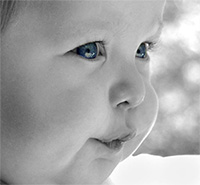
Resize images with the CSS width and height properties
Another way of resizing images is using the CSS width and height properties. Set the width property to a percentage value and the height to "auto". The image is going to be responsive (it will scale up and down).
Example of resizing an image proportionally with the width and height properties:
<!DOCTYPE html>
<html>
<head>
<title>Responsive Resized Image</title>
<style>
img {
width: 100%;
height: auto;
}
</style>
</head>
<body>
<h2>Responsive Resized Image</h2>
<p>Resize the browser to see the responsive effect:</p>
<img src="/uploads/media/default/0001/03/5bfad15a7fd24d448a48605baf52655a5bbe5a71.jpeg" alt="New York" />
</body>
</html>
Resize images with the CSS max-width property
There is a better way for resizing images responsively. If the max-width property is set to 100%, the image will scale down if it has to, but never scale up to be larger than its original size. The trick is to use height: auto; to override any already present height attribute on the image.
Example of adding a responsive resized image with the max-width property:
<!DOCTYPE html>
<html>
<head>
<title>Responsive Resized Image with max-width</title>
<style>
img {
max-width: 100%;
height: auto;
}
</style>
</head>
<body>
<h2>Responsive Resized Image with max-width</h2>
<p>Resize the browser to see the responsive effect:</p>
<img src="/uploads/media/default/0001/03/5bfad15a7fd24d448a48605baf52655a5bbe5a71.jpeg" alt="New York" />
</body>
</html>
Let’s see another live web page example with the same method.
Example of resizing an image proportionally using the max-width property:
<!DOCTYPE html>
<html>
<head>
<title>Responsive Resized Image in a Web Page</title>
<style>
* {
box-sizing: border-box;
}
html {
font-family: "Lucida Sans", sans-serif;
}
img {
width: 100%;
height: auto;
}
.row:after {
content: "";
clear: both;
display: table;
}
.menu,
.content {
float: left;
padding: 15px;
width: 100%;
}
@media only screen and (min-width: 600px) {
.menu {
width: 25%;
}
.content {
width: 75%;
}
}
@media only screen and (min-width: 768px) {
.menu {
width: 20%;
}
.content {
width: 79%;
}
}
.header {
background-color: #1c87c9;
color: #ffffff;
padding: 10px;
text-align: center;
}
.menu ul {
list-style-type: none;
margin: 0;
padding: 0;
}
.menu li {
padding: 8px;
margin-bottom: 7px;
background-color: #666666;
color: #ffffff;
box-shadow: 0 1px 3px rgba(0, 0, 0, 0.12), 0 1px 2px rgba(0, 0, 0, 0.24);
}
.menu li:hover {
background-color: #1c87c9;
}
.footer {
background-color: #1c87c9;
color: #ffffff;
text-align: center;
font-size: 12px;
padding: 15px;
}
</style>
</head>
<body>
<div class="header">
<h1>W3DOCS</h1>
</div>
<div class="row">
<div class="menu">
<ul>
<li>Books</li>
<li>Snippets</li>
<li>Quizzes</li>
<li>Tools</li>
</ul>
</div>
<div class="content">
<h2>About Us</h2>
<p>
W3docs provides free learning materials for programming languages like HTML, CSS, Java Script, PHP etc.
</p>
<p>
It was founded in 2012 and has been increasingly gaining popularity and spreading among both beginners and professionals who want to enhance their programming skills.
</p>
<img src="/uploads/media/default/0001/02/8070c999efd1a155ad843379a5508d16f544aeaf.jpeg" alt="Team Work" />
</div>
</div>
<div class="footer">
<p>
Resize the browser window to see how the content respond to the resizing.
</p>
</div>
</body>
</html>