How to Add an Animated Text Over an Image on Hover With CSS3
It is possible to display an animated text over a faded image on hover using pure CSS.
If you want to mouse over the image and see a text appearing animatedly, then you are in the right place! Let’s see how we can do it step by step.
Create HTML
- Add your image using the <img> tag.
- In a <div> element, put the text you want to appear on hovering.
<h2>Animated Text Over a Faded Image on Hover</h2>
<div class="example">
<img src="/uploads/media/default/0001/01/b408569013c0bb32b2afb0f0d45e93e982347951.jpeg" width="300" height="300" alt="tree" />
<div class="content">
<div class="text">Tree</div>
</div>
</div>
Add CSS
- Set the :hover selector. The hovering effect is set using the :hover pseudo-class that selects and styles the element.
- Set the opacity property. It is the first property you should set, as it specifies the level of transparency of an element. This would hide every element inside the class in the beginning.
- Set the transition property. In the given example, the transition fades the image slowly, in 400 milliseconds. If you do not specify the transition property, there won’t be any effect, and the image will fade quickly.
- Add the transition-delay and transition-duration properties. The first specifies the starting process and the second specifies the time taken from the text to move from top to bottom.
.example {
position: relative;
padding: 0;
width: 300px;
display: block;
cursor: pointer;
overflow: hidden;
}
.content {
opacity: 0;
font-size: 40px;
position: absolute;
top: 0;
left: 0;
color: #1c87c9;
background-color: rgba(200, 200, 200, 0.5);
width: 300px;
height: 300px;
-webkit-transition: all 400ms ease-out;
-moz-transition: all 400ms ease-out;
-o-transition: all 400ms ease-out;
-ms-transition: all 400ms ease-out;
transition: all 400ms ease-out;
text-align: center;
}
.example .content:hover {
opacity: 1;
}
.example .content .text {
height: 0;
opacity: 1;
transition-delay: 0s;
transition-duration: 0.4s;
}
.example .content:hover .text {
opacity: 1;
transform: translateY(250px);
-webkit-transform: translateY(250px);
}
Let’s take the steps with the following example.
Example of creating an animated text going from top to bottom over an image on hover:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
.example {
position: relative;
padding: 0;
width: 300px;
display: block;
cursor: pointer;
overflow: hidden;
}
.content {
opacity: 0;
font-size: 40px;
position: absolute;
top: 0;
left: 0;
color: #1c87c9;
background-color: rgba(200, 200, 200, 0.5);
width: 300px;
height: 300px;
-webkit-transition: all 400ms ease-out;
-moz-transition: all 400ms ease-out;
-o-transition: all 400ms ease-out;
-ms-transition: all 400ms ease-out;
transition: all 400ms ease-out;
text-align: center;
}
.example .content:hover {
opacity: 1;
}
.example .content .text {
height: 0;
opacity: 1;
transition-delay: 0s;
transition-duration: 0.4s;
}
.example .content:hover .text {
opacity: 1;
transform: translateY(250px);
-webkit-transform: translateY(250px);
}
</style>
</head>
<body>
<h2>Animated Text Over a Faded Image on Hover</h2>
<div class="example">
<img src="/uploads/media/default/0001/01/b408569013c0bb32b2afb0f0d45e93e982347951.jpeg" width="300" height="300" alt="tree" />
<div class="content">
<div class="text">Tree</div>
</div>
</div>
</body>
</html>
Result
Animated Text Over a Faded Image on Hover
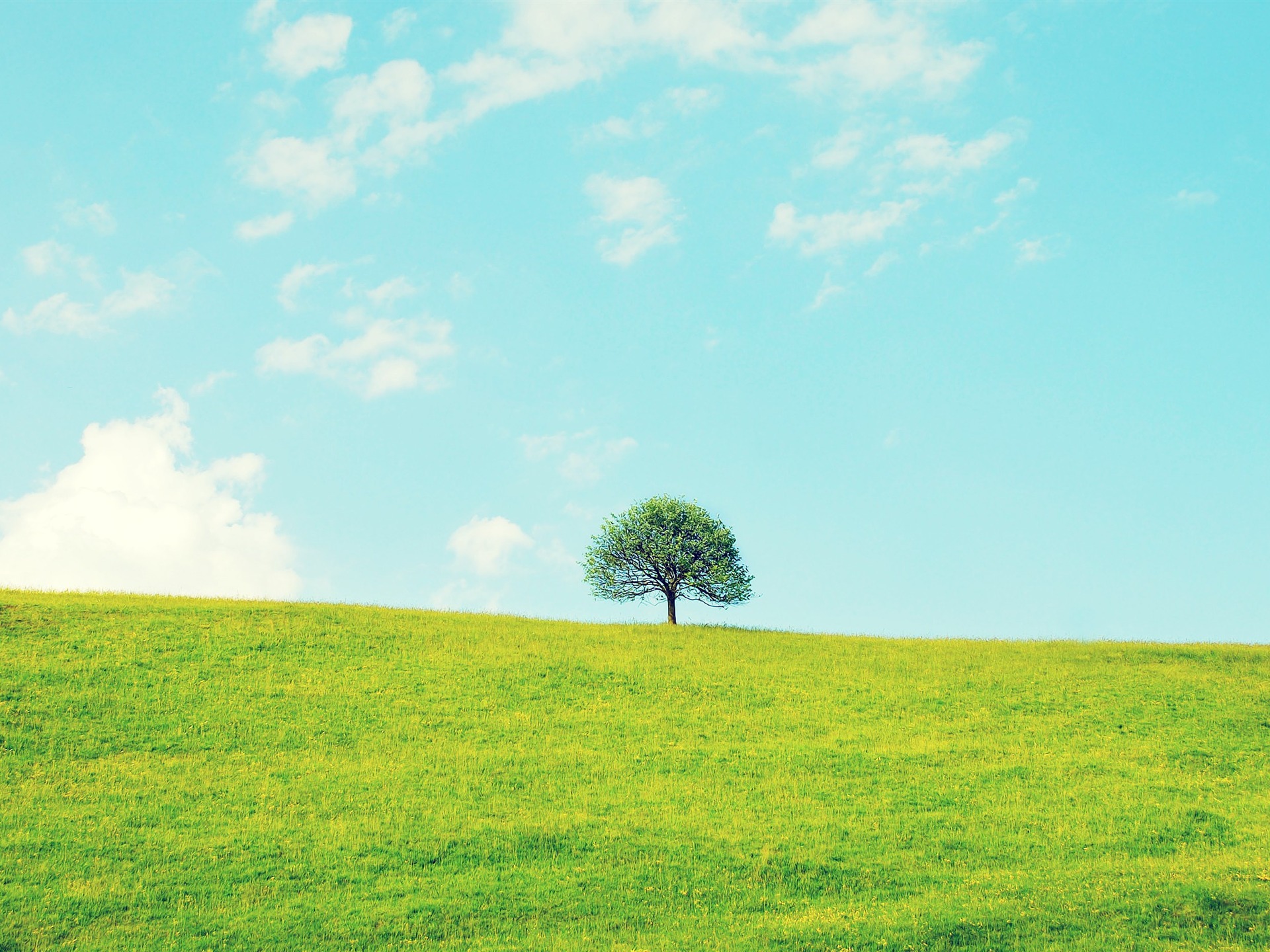
Tree
If you set the text-align property to "left" or "right", the text will show up from the top to bottom on the left and the right sides, respectively.
Example of creating an animated text going from bottom to center over an image on hover:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
.example {
cursor: pointer;
height: 300px;
position: relative;
overflow: hidden;
width: 400px;
text-align: center;
}
.example .fadedbox {
background-color: #666666;
position: absolute;
top: 0;
left: 0;
color: #fff;
-webkit-transition: all 300ms ease-out;
-moz-transition: all 300ms ease-out;
-o-transition: all 300ms ease-out;
-ms-transition: all 300ms ease-out;
transition: all 300ms ease-out;
opacity: 0;
width: 360px;
height: 100px;
padding: 130px 20px;
}
.example:hover .fadedbox {
opacity: 0.8;
}
.example .text {
-webkit-transition: all 300ms ease-out;
-moz-transition: all 300ms ease-out;
-o-transition: all 300ms ease-out;
-ms-transition: all 300ms ease-out;
transition: all 300ms ease-out;
transform: translateY(30px);
-webkit-transform: translateY(30px);
}
.example .title {
font-size: 2.5em;
text-transform: uppercase;
opacity: 0;
transition-delay: 0.2s;
transition-duration: 0.3s;
}
.example:hover .title,
.example:focus .title {
opacity: 1;
transform: translateY(0px);
-webkit-transform: translateY(0px);
}
</style>
</head>
<body>
<h2>Animated Text Over a Faded Image on Hover</h2>
<div class="example">
<img src="/uploads/media/default/0001/01/4982c4f43023330a662b9baed5a407e391ae6161.jpeg" width="400" height="300" alt="house" />
<div class="fadedbox">
<div class="title text"> House </div>
</div>
</div>
</body>
</html>
For maximum browser compatibility, extensions such as -webkit-
for Safari, Google Chrome, and Opera (newer versions), -moz-
for Firefox, -o- for older versions of Opera are used with the transition and transform properties.