The <div> tag is an empty container that is used to define a division or a section. It does not affect the content or layout and is used to group HTML elements to be styled with CSS or manipulated with scripts.
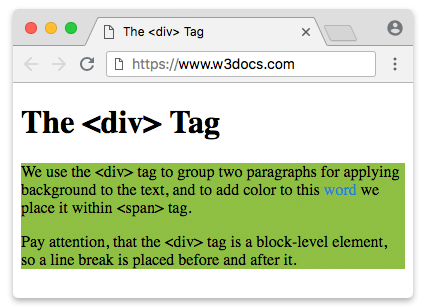
Positioning Blocks Defined by <div> Tag
Since <div> is a block-level element, a line break is placed before and after it.
It is possible to place any HTML element within a <div> tag, including another <div>.
To apply styles inside a paragraph use <span> tag, which is used with inline elements.
Syntax
The <div> tag comes in pairs. The content is written between the opening (<div>) and closing (</div>) tags.
Example of the HTML <div> tag:
<!DOCTYPE html>
<html>
<head>
<title>The <div> Tag</title>
</head>
<body>
<h1> The <div> Tag </h1>
<div style="background-color:#8ebf42">
<p>We use the <div> tag to group two paragraphs for applying a background to the text, and to add color to this
<span style="color:#1c87c9">word</span> we place it within <span> tag.</p>
<p> Pay attention, that the <div> tag is a block-level element, so a line break is placed before and after
it.</p>
</div>
</body>
</html>
When we build layouts, we deal with multiple blocks defined by the <div> tag. The positioning of these blocks is at the heart of layout: placing elements in the correct relative positions across all screen sizes is one of the most important tasks.
Depending on the content and the goals of the page, we can use different techniques (or their combinations) to determine the place of each block. Let’s learn more about these techniques.
Flexbox ¶
In modern websites float-based layouts are being replaced with Flexbox. The main idea behind Flexbox is that with it, you can control the alignment, direction, order, and size of the items inside the container.
Example of a Flexbox with HTML <div> tag:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
.flex-container {
display: flex;
align-items: center; /* Use another value to see the result */
width: 100%;
height: 200px;
background-color: #1faadb;
}
.flex-container > div {
width: 25%;
height: 60px;
margin: 5px;
border-radius: 3px;
background-color: #8ebf42;
}
</style>
</head>
<body>
<div class="flex-container">
<div></div>
<div></div>
<div></div>
</div>
</body>
</html>
CSS Float property ¶
CSS float property, or "floats" allows elements to appear next to, or apart from, one another, which lets us create different types of layouts, including multi-column pages, sidebars, grids, etc.
Example of the HTML <div> tag with the CSS float property:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
.content {
overflow: auto;
border: 3px solid #666;
}
.content div {
padding: 10px;
}
.content a {
color: darkblue;
}
.blue {
float: right;
width: 45%;
background-color: #1faadb;
}
.green {
float: left;
width: 35%;
background-color: #8ebf42;
}
</style>
</head>
<body>
<div class="content">
<div class="blue">
<p>This is some paragraph inside div tag.</p>
<a href="#">This is some hyperlink inside div tag.</a>
<ul>
<li>List item 1</li>
<li>List item 2</li>
</ul>
</div>
<div class="green">
<p>This is some paragraph inside div tag.</p>
<ol>
<li>List item 1</li>
<li>List item 2</li>
</ol>
</div>
</div>
</body>
</html>
Result
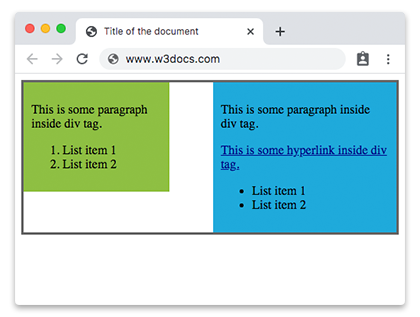
Negative Margins ¶
Negative margins can be applied to both static or floated elements.
Example of negative margins:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
.content div {
padding: 2%;
}
.content a {
color: darkblue;
}
.main-content {
width: 30%;
margin-left: 32%;
}
.blue {
width: 25%;
margin-top: -10%;
background-color: #1faadb;
}
.green {
width: 20%;
margin: -35% auto auto 70%;
background-color: #8ebf42;
}
</style>
</head>
<body>
<div class="content">
<div class="main-content">
<a href="#">This is some hyperlink inside div tag.</a>
</div>
<div class="blue">
<p>This is some paragraph inside div tag.</p>
<a href="#">This is some hyperlink inside div tag.</a>
<ul>
<li>List item 1</li>
<li>List item 2</li>
</ul>
</div>
<div class="green">
<p>This is some paragraph inside div tag.</p>
<ol>
<li>List item 1</li>
<li>List item 2</li>
</ol>
</div>
</div>
</body>
</html>
Relative+absolute positioning ¶
If you want to position div relative to particular element you can use a combination of position: relative and position: absolute.
Example of relative+absolute positioning of HTML <div> tag:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
.content {
position: relative;
height: 400px;
border: 1px solid #666;
}
.content div {
position: absolute;
width: 35%;
padding: 10px;
}
.blue {
right: 20px;
bottom: 0;
background-color: #1faadb;
}
.green {
top: 10px;
left: 15px;
background-color: #8ebf42;
}
</style>
</head>
<body>
<div class="content">
<div class="blue">
<p>This is some paragraph inside div tag.</p>
</div>
<div class="green">
<p>This is some paragraph inside div tag.</p>
</div>
</div>
</body>
</html>
Attributes ¶
Attribute | Value | Description |
---|---|---|
align | left right center justify |
Was used to align the content inside a <div> tag. This attribute is not supported in HTML5. CSS text-align property is used instead. |
The <div> tag also supports the Global attributes and the Event Attributes.
How to style <div> tag?
Common properties to alter the visual weight/emphasis/size of text in <div> tag:
- CSS font-style property sets the style of the font. normal | italic | oblique | initial | inherit.
- CSS font-family property specifies a prioritized list of one or more font family names and/or generic family names for the selected element.
- CSS font-size property sets the size of the font.
- CSS font-weight property defines whether the font should be bold or thick.
- CSS text-transform property controls text case and capitalization.
- CSS text-decoration property specifies the decoration added to text, and is a shorthand property for text-decoration-line, text-decoration-color, text-decoration-style.
Coloring text in <div> tag:
- CSS color property describes the color of the text content and text decorations.
- CSS background-color property sets the background color of an element.
Text layout styles for <div> tag:
- CSS text-indent property specifies the indentation of the first line in a text block.
- CSS text-overflow property specifies how overflowed content that is not displayed should be signalled to the user.
- CSS white-space property specifies how white-space inside an element is handled.
- CSS word-break property specifies where the lines should be broken.
Other properties worth looking at for <div> tag:
- CSS text-shadow property adds shadow to text.
- CSS text-align-last property sets the alignment of the last line of the text.
- CSS line-height property specifies the height of a line.
- CSS letter-spacing property defines the spaces between letters/characters in a text.
- CSS word-spacing property sets the spacing between words.
Browser support
|
|
|
|
|
---|---|---|---|---|
✓ | ✓ | ✓ | ✓ | ✓ |
Practice Your Knowledge
Quiz Time: Test Your Skills!
Ready to challenge what you've learned? Dive into our interactive quizzes for a deeper understanding and a fun way to reinforce your knowledge.