Introduction to the Storage API
In modern web development, managing data efficiently is crucial. JavaScript's Storage API provides powerful mechanisms for storing data directly in the browser, which can be leveraged for enhancing user experiences and building complex applications. This guide explores the essentials of the Storage API, focusing primarily on localStorage
and sessionStorage
.
Understanding localStorage
localStorage
is a part of the web storage that allows storing data with no expiration date. This data persists even after the browser window is closed, making it ideal for data that needs to persist across sessions.
Storing Data in localStorage
To store data using localStorage
, you can use the setItem
method. Here's a simple example:
// Storing data in localStorage
localStorage.setItem('username', 'JohnDoe');
This script stores a username in the browser's local storage. The data will remain available for future sessions.
Retrieving Data from localStorage
To retrieve data, the getItem
method is used. Here's how you can fetch the previously stored username:
// Retrieving data from localStorage
var username = localStorage.getItem('username');
console.log(username); // Outputs: JohnDoe
Removing Data from localStorage
You can remove data from localStorage
using the removeItem
method:
// Removing data from localStorage
localStorage.removeItem('username');
This method deletes the specific key from the storage, ensuring that it's no longer accessible.
Leveraging sessionStorage
sessionStorage
is similar to localStorage
but with a shorter lifespan. Data stored in sessionStorage
is cleared when the page session ends (i.e., when the browser tab is closed).
Storing Data in sessionStorage
Here is how you can store data in sessionStorage
:
// Storing data in sessionStorage
sessionStorage.setItem('sessionName', 'Session1');
This code snippet stores a session name that will only be available as long as the browser tab remains open.
Retrieving Data from sessionStorage
To retrieve the stored data from sessionStorage
, use the getItem
method:
// Retrieving data from sessionStorage
var sessionName = sessionStorage.getItem('sessionName');
console.log(sessionName); // Outputs: Session1
Removing Data from sessionStorage
Similarly, to remove data, use the removeItem
method:
// Removing data from sessionStorage
sessionStorage.removeItem('sessionName');
Best Practices for Using Web Storage
- Security: Always consider the security implications of storing sensitive data in the browser. Avoid storing confidential information such as passwords or personal identification details.
- Storage Limits: Be mindful of the storage limitations (usually about 5MB) and handle cases where the storage might be full.
- Cross-Browser Compatibility: Ensure your code handles scenarios where a browser may not support the storage APIs.
A Full Example to Grasp it All
This demo showcases how to use the Web Storage API, including both localStorage
and sessionStorage
. You have buttons to store, retrieve, and remove data from storage:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>Storage API Interactive Demo</title>
</head>
<body>
<h2>localStorage and sessionStorage Demo</h2>
<div style="display: flex; gap: 10px">
<button onclick="storeInLocal()">Store in localStorage</button>
<button onclick="retrieveFromLocal()">Retrieve from localStorage</button>
<button onclick="removeFromLocal()">Remove from localStorage</button>
</div>
<div style="margin: 20px 0" id="localStorageResult"></div>
<div style="display: flex; gap: 10px; margin-top: 10px">
<button onclick="storeInSession()">Store in sessionStorage</button>
<button onclick="retrieveFromSession()">
Retrieve from sessionStorage
</button>
<button onclick="removeFromSession()">Remove from sessionStorage</button>
</div>
<div style="margin-top: 20px" id="sessionStorageResult"></div>
<script>
function storeInLocal() {
localStorage.setItem("demo", "Hi form LocalStorage!");
document.getElementById("localStorageResult").textContent =
"Stored in localStorage: " + localStorage.getItem("demo");
}
function retrieveFromLocal() {
const value = localStorage.getItem("demo") || "Nothing in localStorage";
document.getElementById("localStorageResult").textContent =
"Retrieved from localStorage: " + value;
}
function removeFromLocal() {
localStorage.removeItem("demo");
document.getElementById("localStorageResult").textContent =
"Item removed from localStorage.";
}
function storeInSession() {
sessionStorage.setItem("demo", "Hi from SessionStorage!");
document.getElementById("sessionStorageResult").textContent =
"Stored in sessionStorage: " + sessionStorage.getItem("demo");
}
function retrieveFromSession() {
const value =
sessionStorage.getItem("demo") || "Nothing in sessionStorage";
document.getElementById("sessionStorageResult").textContent =
"Retrieved from sessionStorage: " + value;
}
function removeFromSession() {
sessionStorage.removeItem("demo");
document.getElementById("sessionStorageResult").textContent =
"Item removed from sessionStorage.";
}
</script>
</body>
</html>
- LocalStorage: Data stored here remains even after the browser is closed and reopened, making it perfect for saving user preferences or other long-term data.
- SessionStorage: This is similar to localStorage but the data is cleared when the session ends (like when the browser is closed).
By clicking on the various buttons, you can see how data is added to, retrieved from, and removed from each type of storage. The results are displayed directly below each button, giving you immediate feedback on what is happening with the data in storage. This interactive setup helps you visualize and understand how web applications can remember data between page reloads or browser sessions.
In addition to interacting with the storage operations through the buttons in this demo, you can also view and manage the stored data directly in your web browser. Open your browser's developer tools, navigate to the Application section, and then look under the Storage tab. Here, you can see localStorage
and sessionStorage
entries.
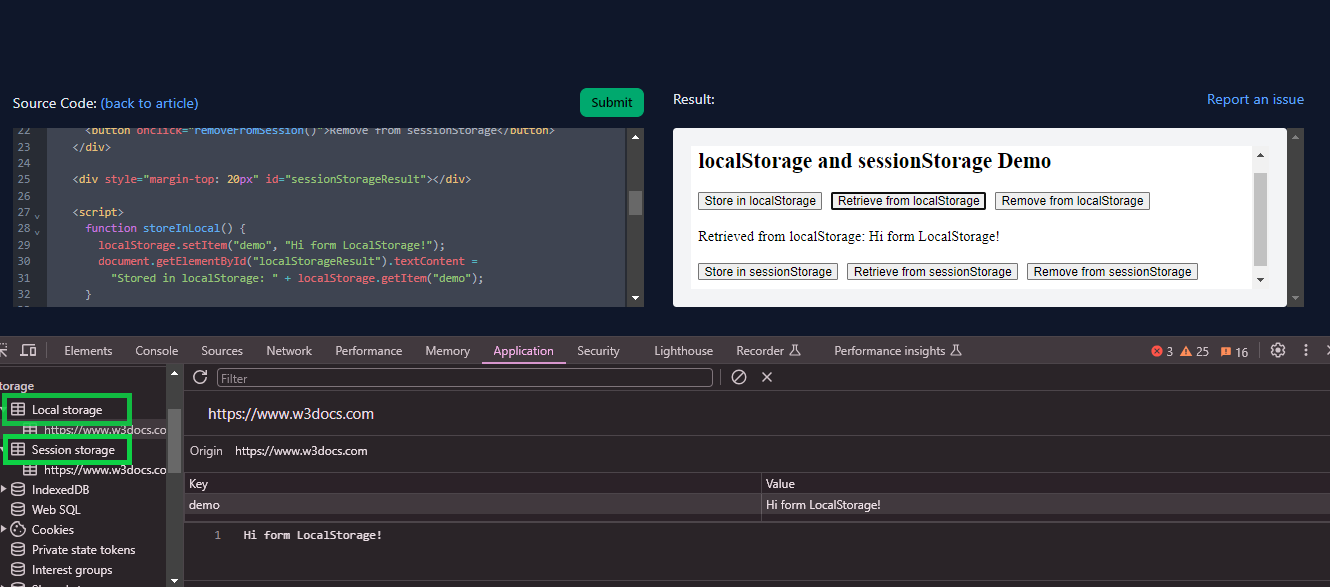
This visual tool allows you to see the effects of your actions (like storing and removing data) in real-time and provides a practical way to explore how web storage works in browsers.
Conclusion
The JavaScript Storage API provides a robust and easy-to-use method to manage data within the browser. By understanding and leveraging localStorage
and sessionStorage
, developers can significantly enhance the user experience of their web applications. Always consider security and storage limits to ensure your applications are robust and user-friendly. With these tools, you can create persistent states and data management features that are critical for modern web applications.
Practice Your Knowledge
Quiz Time: Test Your Skills!
Ready to challenge what you've learned? Dive into our interactive quizzes for a deeper understanding and a fun way to reinforce your knowledge.