In the world of web development, mastering JavaScript is a critical step toward creating dynamic and interactive web applications. Among its powerful features, IndexedDB stands out as an essential tool for web developers aiming to manage client-side storage of significant amounts of structured data. This article delves deep into the nuances of IndexedDB, providing you with the knowledge and code examples necessary to leverage this feature in your JavaScript projects.
Understanding IndexedDB in JavaScript
IndexedDB is a low-level API for client-side storage of significant amounts of structured data, including files/blobs. This API uses indexes to enable high-performance searches of this data. Unlike localStorage, which allows you to store only simple key/value pairs, IndexedDB supports the storage of more complex data types.
Key Features of IndexedDB:
- Asynchronous: IndexedDB operations do not block the main thread, ensuring smooth UI experiences.
- Large Storage Capacity: It can store a significant amount of data, far more than cookies or localStorage.
- Support for Complex Data Types: It allows you to store almost any type of value by using JavaScript objects.
Getting Started with IndexedDB
To start using IndexedDB, you need to understand its basic concepts including databases, object stores, and transactions.
Opening a Database
Opening an IndexedDB database is the first step to working with data. Here’s how you can do it:
let dbRequest = window.indexedDB.open("MyTestDatabase", 1);
dbRequest.onerror = function(event) {
// Handle errors.
};
dbRequest.onsuccess = function(event) {
let db = dbRequest.result;
// Perform operations on the database.
};
This code snippet attempts to open a database named "MyTestDatabase". The open
method takes two parameters: the name of the database and the version. If the database does not exist, it will be created.
Creating an Object Store
After opening the database, the next step is to create an object store. Think of an object store as a table in a relational database, but more flexible.
dbRequest.onupgradeneeded = function(event) {
let db = event.target.result;
let objectStore = db.createObjectStore("name", { keyPath: "id" });
// Create an index to search customers by name.
objectStore.createIndex("name", "name", { unique: false });
// Create an index to search customers by email.
objectStore.createIndex("email", "email", { unique: true });
};
This code creates an object store with a keyPath
of "id", meaning the store uses the "id" property of its objects as the key. It also creates two indexes, one for searching by name and another for email.
Working with Data
With the database and object store set up, you can start performing operations such as adding, retrieving, and deleting data.
Adding Data
function addData(db) {
let transaction = db.transaction(["name"], "readwrite");
let objectStore = transaction.objectStore("name");
let request = objectStore.add({ id: "1", name: "John Doe", email: "[email protected]" });
request.onsuccess = function(event) {
// Data added successfully
};
request.onerror = function(event) {
// Handle errors.
};
}
This function adds a new object to the "name" object store. It creates a transaction, specifies the object store, and then adds the data.
Retrieving Data
function getData(db, key) {
let transaction = db.transaction(["name"]);
let objectStore = transaction.objectStore("name");
let request = objectStore.get(key);
request.onsuccess = function(event) {
if (request.result) {
console.log("Name: ", request.result.name);
// Perform operations on the result.
} else {
console.log("No data found with the given key.");
}
};
}
This function retrieves data from the "name" object store using a specified key. If the data exists, it logs the name to the console.
An Interactive Example
Let's create an interactive example using IndexedDB that demonstrates basic operations like creating a database, adding records, and retrieving them. This example will involve a simple task list where users can add and view their tasks.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>IndexedDB Example</title>
</head>
<body>
<h1>Task Manager</h1>
<input type="text" id="taskInput" placeholder="Enter a task" />
<button onclick="addTask()">Add Task</button>
<ul id="taskList"></ul>
<script>
let db;
window.onload = function () {
let request = window.indexedDB.open("TaskDB", 1);
request.onerror = function (event) {
console.error("Database error:", event.target.errorCode);
};
request.onsuccess = function (event) {
db = request.result;
readTasks();
};
request.onupgradeneeded = function (event) {
let db = event.target.result;
let objectStore = db.createObjectStore("tasks", {
keyPath: "id",
autoIncrement: true,
});
objectStore.createIndex("task", "task", { unique: false });
};
};
function addTask() {
let task = document.getElementById("taskInput").value;
let transaction = db.transaction(["tasks"], "readwrite");
let objectStore = transaction.objectStore("tasks");
let request = objectStore.add({ task: task });
request.onsuccess = function () {
readTasks();
};
request.onerror = function (event) {
console.error("Error adding task:", event.target.errorCode);
};
document.getElementById("taskInput").value = "";
}
function readTasks() {
let objectStore = db.transaction("tasks").objectStore("tasks");
document.getElementById("taskList").innerHTML = "";
objectStore.openCursor().onsuccess = function (event) {
let cursor = event.target.result;
if (cursor) {
let li = document.createElement("li");
li.textContent = cursor.value.task;
document.getElementById("taskList").appendChild(li);
cursor.continue();
}
};
}
</script>
</body>
</html>
How It Works:
- Database Setup: When the page loads, the script opens a database called
TaskDB
. If it doesn't exist, it creates one and sets up an object store namedtasks
. - Adding Tasks: Users can enter tasks in the input box. When the "Add Task" button is clicked, the task is stored in the IndexedDB.
- Reading Tasks: Every time a task is added, the list of tasks is updated. This is done by reading all entries in the
tasks
object store and displaying them as list items in the HTML.
This example provides a practical demonstration of how to use IndexedDB to manage a simple task list in a web application. It shows the basics of setting up a database, adding data, and reading data back from the store.
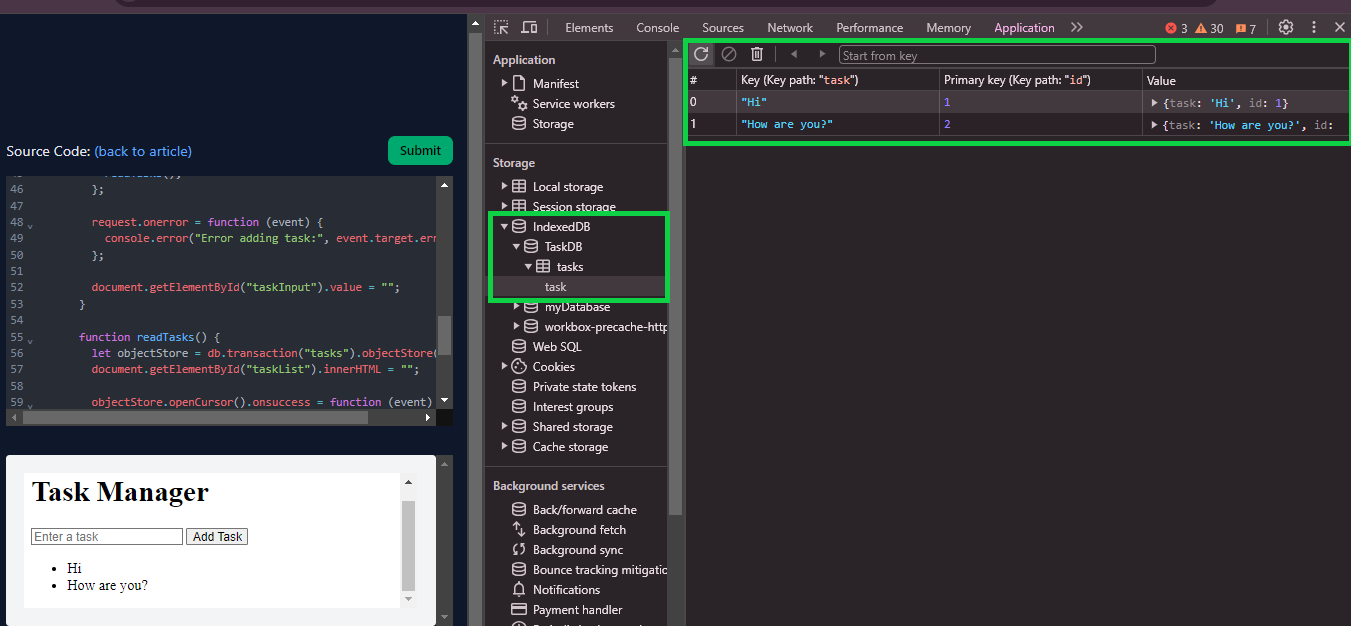
To view the results of your interactions with IndexedDB directly in your browser, you can inspect the stored data within the browser's developer tools:
- Open Developer Tools: Use
Ctrl+Shift+I
(orCmd+Option+I
on Mac) to open Developer Tools in most browsers like Chrome, Firefox, or Edge. - Navigate to Storage: Look for the "Application" tab in Chrome (or "Storage" tab in Firefox).
- Find IndexedDB: Inside the Application or Storage tab, you will see a section labeled "IndexedDB". Click on it to expand and view the databases.
- Inspect the Database: Click on the name of your database (
TaskDB
in the example). You should see the object store (tasks
), where you can click to view all the stored records.
This allows you to see all data entries, modify them, or even clear the database during testing, providing a hands-on way to understand how data is managed within your application.
Conclusion
IndexedDB is a powerful feature in JavaScript that enables web developers to store and manage significant amounts of structured data on the client side. By understanding and utilizing its capabilities, developers can create more sophisticated and performant web applications. The code examples provided in this guide serve as a starting point for integrating IndexedDB into your JavaScript projects. Experiment with these snippets, and explore the API further to fully harness the power of IndexedDB in your applications.
Practice Your Knowledge
Quiz Time: Test Your Skills!
Ready to challenge what you've learned? Dive into our interactive quizzes for a deeper understanding and a fun way to reinforce your knowledge.