How to Position One Image on Top of Another in HTML/CSS
Sometimes, you may need to position one image on top of another. This can be easily done with HTML and CSS. For that, you can use the CSS position and z-index properties.
First, we are going to show an example where we use the position property.
Create HTML
- Use a <div> with a class name "parent".
- Add two <img> elements with the following class names: “image1” and “image2”.
The first image will be the background image and the second will overlay it.
<div class="parent">
<img class="image1" src="/uploads/media/default/0001/01/25acddb3da54207bc6beb5838f65f022feaa81d7.jpeg" />
<img class="image2" src="/uploads/media/default/0001/01/25acddb3da54207bc6beb5838f65f022feaa81d7.jpeg" />
</div>
Add CSS
- Add a relative div placed in the flow of the page.
- Set the background image as relative so as the div knows how big it must be.
- Set the overlay as absolute, which will be relative to the upper-left edge of the container div.
We place the background image at the beginning of the container, and then we will set the overlay image to start from 30 pixels after the background.
.parent {
position: relative;
top: 0;
left: 0;
}
.image1 {
position: relative;
top: 0;
left: 0;
border: 1px solid #000000;
}
.image2 {
position: absolute;
top: 30px;
left: 30px;
border: 1px solid #000000;
}
Let’s see the full example.
Example of positioning an image on top of another using the position property:
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
.parent {
position: relative;
top: 0;
left: 0;
}
.image1 {
position: relative;
top: 0;
left: 0;
border: 1px solid #000000;
}
.image2 {
position: absolute;
top: 30px;
left: 30px;
border: 1px solid #000000;
}
</style>
</head>
<body>
<div class="parent">
<img class="image1" src="/uploads/media/default/0001/01/25acddb3da54207bc6beb5838f65f022feaa81d7.jpeg" />
<img class="image2" src="/uploads/media/default/0001/01/25acddb3da54207bc6beb5838f65f022feaa81d7.jpeg" />
</div>
</body>
</html>
Result
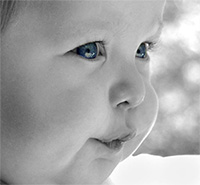
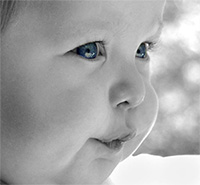
In this example, we used both the relative and absolute values of the position property. The relative value places an element relative to its normal position. The absolute value removes elements from the document flow, and elements are positioned relative to its parent element's position.
Now, let’s see an example where we use both the position and z-index properties.
Example of positioning an image on top of another using the position and z-index properties:
The z-index is a CSS property to determine which element to overlay and which element to be in the background. The idea is to imagine a z-axis perpendicular to the screen. The higher the z-index of an element, the element will be shown nearer to the one viewing the page, thus overlaying the other elements with a lower z-index.
<!DOCTYPE html>
<html>
<head>
<title>Title of the document</title>
<style>
.image1 {
position: absolute;
top: 10px;
left: 10px;
border: 1px solid #000000;
z-index: 1;
}
.image2 {
position: absolute;
top: 25px;
left: 25px;
border: 1px solid #000000;
z-index: 2;
}
</style>
</head>
<body>
<img class="image1" src="/uploads/media/default/0001/01/25acddb3da54207bc6beb5838f65f022feaa81d7.jpeg" />
<img class="image2" src="/uploads/media/default/0001/01/25acddb3da54207bc6beb5838f65f022feaa81d7.jpeg" />
</body>
</html>
In the example mentioned above, we set the position to absolute for both elements. You can try the snippet yourself, and see that you can swap the overlaying and the background image easily by changing the z-index. By z-index you can set any element on top of another one, and not just images.